Temperature indicator using 7segment display & Arduino Nano
- Ramesh G
- Mar 5, 2022
- 3 min read
In this tutorial, you will learn how you can test TM1637 4-digit 7-segment displays with Arduino and Temperature sensor DHT11 interface.
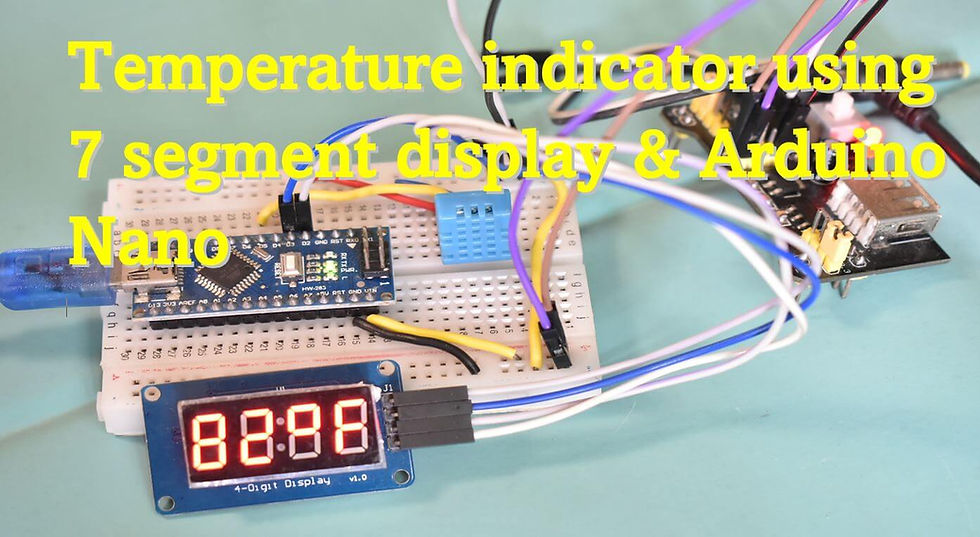
Components required
Arduino Nano - 1 no
TM1637 module - 1 no
DHT 11 - 1 no
5V/3.3V Power module - 1 no
Circuit diagram
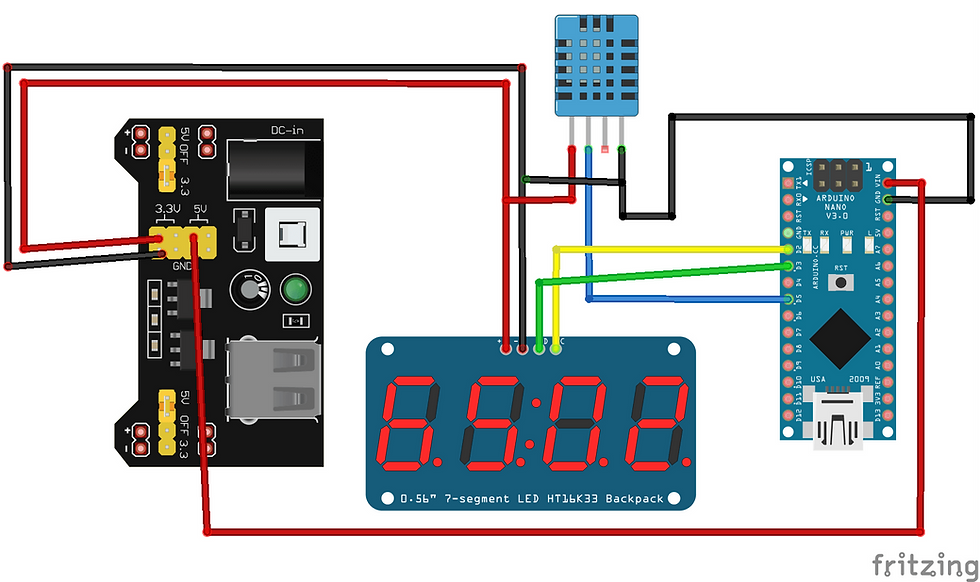
TM1637
TM1637 seven segment modules is a ready made multiplexed seven segment display with 4 digits. The driver IC is TM1637; It has 4pin control there are GND, VCC, DIO, CLK. only two signal lines can make MCU control four Digit 7-segments LED can be used to display decimal, letters and so on. The 7segment LED Display has common anode type.
TM1637 4-Digit 7-Segment Display Specifications
Operating voltage 3.3 – 5 V
Current draw-80 mA
Operating temperature-10 – 80 °C
Interfacing TM1637 with the arduino:
So the first thing you want to do is connect the clock pin to any pin on the Arduino.
CLK clock pin of the TM1637 with the digital pin 3 of the arduno.
DIO pin of the TM1637 with digital pin 2 of the arduino.
VCC of the TM1637 with the 5V of the arduino.
Gnd of the TM1637 with the ground of the arduino.
DHT11
Connecting DHT11/DHT22/AM2302 sensor to Arduino nano is fairly simple.
Connect VCC pin on the sensor to the 3.3V pin on the Arduino nano and ground to ground. Also connect Data pin on the sensor to D5 pin of the Arduino nano.
Installing Library
7segment: you need to Download and install the TM1637 library.
Temperature sensor: you need to Download and install the DHT11 library.
Follow the next steps to install those libraries.
In your Arduino IDE, to install the libraries go to Sketch > Include Library > Add .ZIP library… and select the library you’ve just downloaded.
After installing the required libraries, copy the following code to your Arduino IDE.
Arduino Code
First testing the TM1637 display check.
#include <Arduino.h>
#include <TM1637Display.h>
// Module connection pins (Digital Pins)
#define CLK 2
#define DIO 3
// The amount of time (in milliseconds) between tests
#define TEST_DELAY 500
const uint8_t SEG_DONE[] = {
SEG_B | SEG_C | SEG_D | SEG_E | SEG_G, // d
SEG_D | SEG_C | SEG_F | SEG_E | SEG_G, // b
SEG_C | SEG_D | SEG_E | SEG_G, // O
SEG_D | SEG_E | SEG_F | SEG_G, // t
};
TM1637Display display(CLK, DIO);
void setup()
{
}
void loop()
{
int k;
uint8_t data[] = { 0xff, 0xff, 0xff, 0xff };
uint8_t blank[] = { 0x00, 0x00, 0x00, 0x00 };
display.setBrightness(0x0f);
// All segments on
display.setSegments(data);
delay(TEST_DELAY);
// Selectively set different digits
data[0] = display.encodeDigit(0);
data[1] = display.encodeDigit(1);
data[2] = display.encodeDigit(2);
data[3] = display.encodeDigit(3);
display.setSegments(data);
delay(TEST_DELAY);
/*
for(k = 3; k >= 0; k--) {
display.setSegments(data, 1, k);
delay(TEST_DELAY);
}
*/
display.clear();
display.setSegments(data+2, 2, 2);
delay(TEST_DELAY);
display.clear();
display.setSegments(data+2, 2, 1);
delay(TEST_DELAY);
display.clear();
display.setSegments(data+1, 3, 1);
delay(TEST_DELAY);
// Show decimal numbers with/without leading zeros
display.showNumberDec(0, false); // Expect: ___0
delay(TEST_DELAY);
display.showNumberDec(0, true); // Expect: 0000
delay(TEST_DELAY);
display.showNumberDec(1, false); // Expect: ___1
delay(TEST_DELAY);
display.showNumberDec(1, true); // Expect: 0001
delay(TEST_DELAY);
display.showNumberDec(301, false); // Expect: _301
delay(TEST_DELAY);
display.showNumberDec(301, true); // Expect: 0301
delay(TEST_DELAY);
display.clear();
display.showNumberDec(14, false, 2, 1); // Expect: _14_
delay(TEST_DELAY);
display.clear();
display.showNumberDec(4, true, 2, 2); // Expect: __04
delay(TEST_DELAY);
display.showNumberDec(-1, false); // Expect: __-1
delay(TEST_DELAY);
display.showNumberDec(-12); // Expect: _-12
delay(TEST_DELAY);
display.showNumberDec(-999); // Expect: -999
delay(TEST_DELAY);
display.clear();
display.showNumberDec(-5, false, 3, 0); // Expect: _-5_
delay(TEST_DELAY);
display.showNumberHexEx(0xf1af); // Expect: f1Af
delay(TEST_DELAY);
display.showNumberHexEx(0x2c); // Expect: __2C
delay(TEST_DELAY);
display.showNumberHexEx(0xd1, 0, true); // Expect: 00d1
delay(TEST_DELAY);
display.clear();
display.showNumberHexEx(0xd1, 0, true, 2); // Expect: d1__
delay(TEST_DELAY);
// Run through all the dots
for(k=0; k <= 4; k++) {
display.showNumberDecEx(0, (0x80 >> k), true);
delay(TEST_DELAY);
}
// Brightness Test
for(k = 0; k < 4; k++)
data[k] = 0xff;
for(k = 0; k < 7; k++) {
display.setBrightness(k);
display.setSegments(data);
delay(TEST_DELAY);
}
// On/Off test
for(k = 0; k < 4; k++) {
display.setBrightness(7, false); // Turn off
display.setSegments(data);
delay(TEST_DELAY);
display.setBrightness(7, true); // Turn on
display.setSegments(data);
delay(TEST_DELAY);
}
// Done!
display.setSegments(SEG_DONE);
while(1);
}

Next Temperature indication
// Include the libraries
#include <TM1637Display.h>
#include <Adafruit_Sensor.h>
#include <DHT.h>
// Define the connections pins
#define CLK 2
#define DIO 3
#define DHTPIN 5
// Create variable
int temperature_celsius;
int temperature_fahrenheit;
// Create °C symbol
const uint8_t celsius[] = {
SEG_A | SEG_B | SEG_F | SEG_G, // Circle
SEG_A | SEG_D | SEG_E | SEG_F // C
};
// Create °F symbol
const uint8_t fahrenheit[] = {
SEG_A | SEG_B | SEG_F | SEG_G, // Circle
SEG_A | SEG_E | SEG_F | SEG_G // F
};
#define DHTTYPE DHT11 // DHT 11
//#define DHTTYPE DHT22 // DHT 22 (AM2302)
// Create display object of type TM1637Display
TM1637Display display = TM1637Display(CLK, DIO);
// Create dht object of type DHT:
DHT dht = DHT(DHTPIN, DHTTYPE);
void setup() {
// Set the display brightness (0-7)
display.setBrightness(5);
// Clear the display
display.clear();
// Setup sensor
dht.begin();
}
void loop() {
// Read the temperature as Celsius and Fahrenheit
temperature_celsius = dht.readTemperature();
temperature_fahrenheit = dht.readTemperature(true);
// Display the temperature in celsius format
display.showNumberDec(temperature_celsius, false, 2, 0);
display.setSegments(celsius, 2, 2);
delay(1000);
// Display the temperature in fahrenheit format
display.showNumberDec(temperature_fahrenheit, false, 2, 0);
display.setSegments(fahrenheit, 2, 2);
delay(1000);
}
Demo
Comments