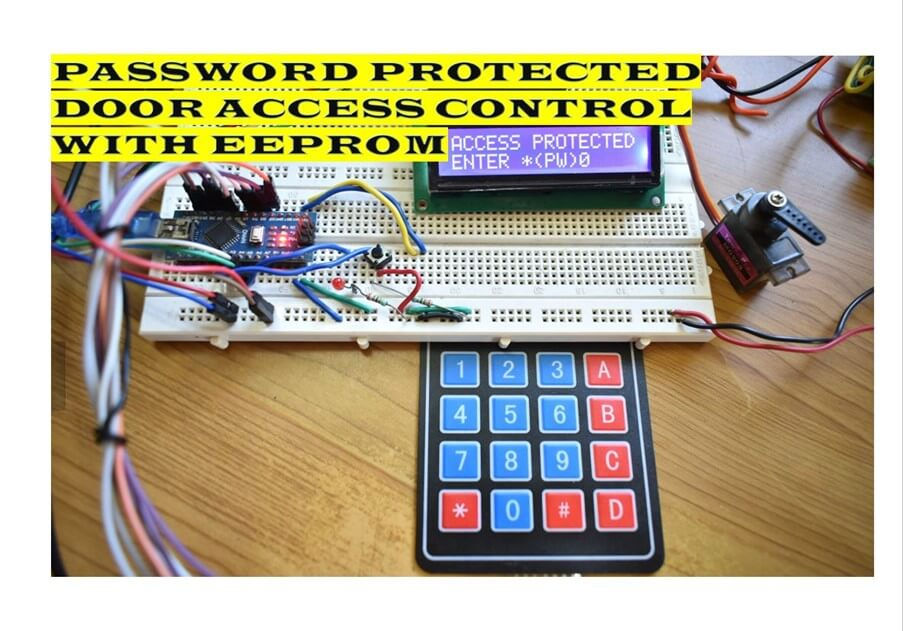
Here to learn How to Make an password protected Door Opening System Using Arduino, LCD, servo, Push button, LED and Matrix keypad. The Red LED using for Door opening indication and Push button used emergency bypass for secured password protected door opening in case of you forget the password.
The password storing in Arduino EEPROM and you can change the password any time and values stored in EEPROM. When the power of the Arduino the EEPROM remember the previously stored password.
Circuit Diagram
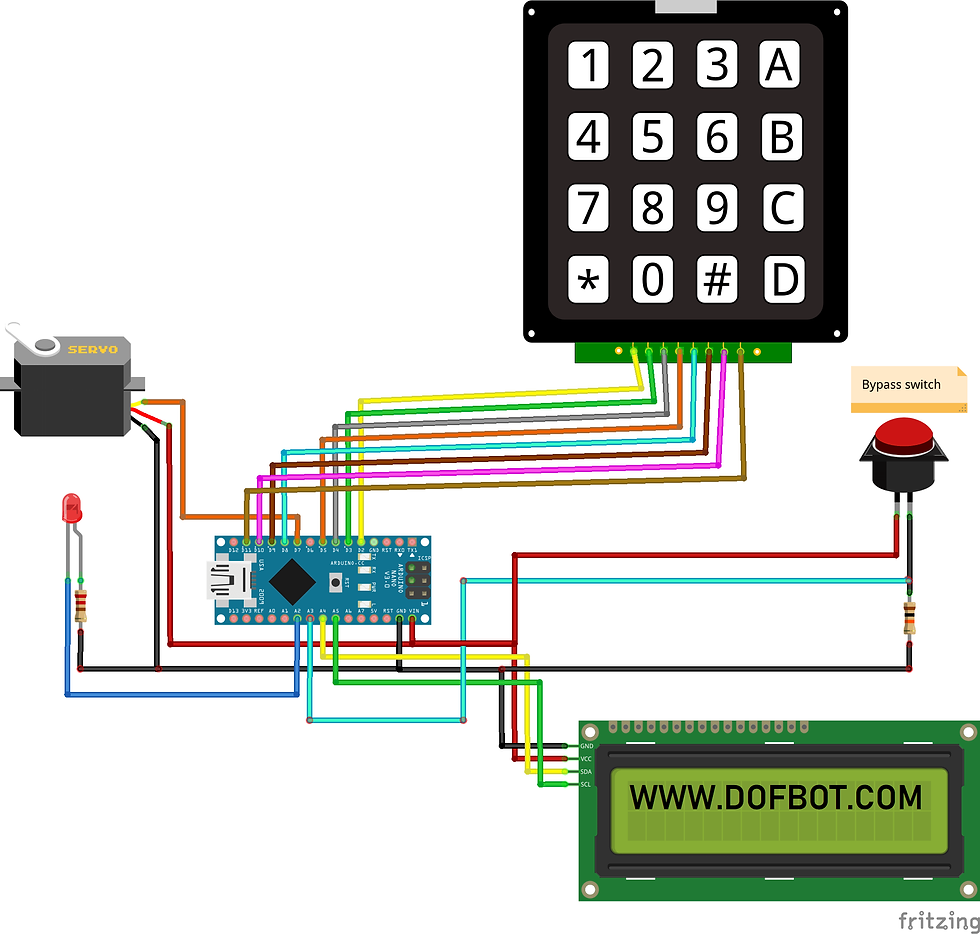
Components Required
Arduino Nano - 1 no
LCD 16x2 with I²C module - 1no
4x4 Matrix keypad - 1no
Red LED - 1 no
Servo mini SG90 - 1 no
Push Button - 1no
10k resistor- 1no
Servo motor
A servo motor is an electric device used for precise control of angular rotation. It is used in applications that demand precise control over motion, like in case of control of a robotic arm.
The rotation angle of the servo motor is controlled by applying a PWM signal to it.
By varying the width of the PWM signal, we can change the rotation angle and direction of the motor.
Wire Configuration:
Brown - Ground wire connected to the ground of system
Red - Powers the motor typically +5V is used
Orange - PWM signal is given in through this wire to drive the motor
Servo SG90 Data Sheet
4x4 keypad module
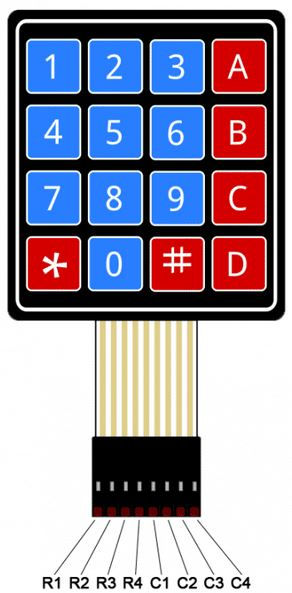
4x4 keypad will have eight terminals. In them four are rows of matrix and four are columns of matrix. These 8 pins are driven out from 16 buttons present in the module. Those 16 alphanumeric digits on the module surface are the 16 buttons arranged in matrix formation.
Using keypad module is little tricky. As 16 keys are connected in matrix formation the module is a little complex to use. The module gives only 8 pins as a way for interacting with 16 buttons.
We are going to explain how to use the keypad module in a simple way step by step:
Consider we have connected the keypad module to a microcontroller.
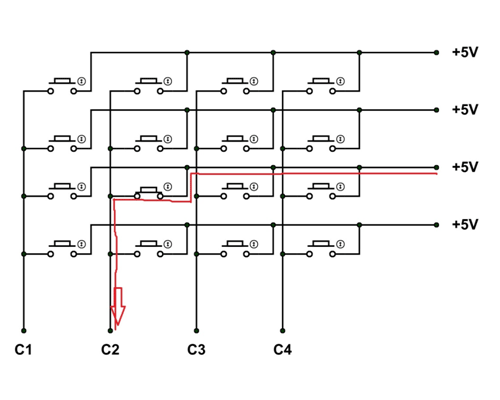
Step1: first set all rows to output and set them at +5v. Next set all columns as input to sense the high logic. Now consider a button is pressed on keypad. And that key is at 2nd column and 3rd row.
With the button being pressed the current flows as shown in figure. With that a voltage of +5v appears at terminal c2. Since the column pins are set as inputs, the controller can sense c2 going high. The controller can be programmed to remember that c2 going high and the button pressed is in c2 column.
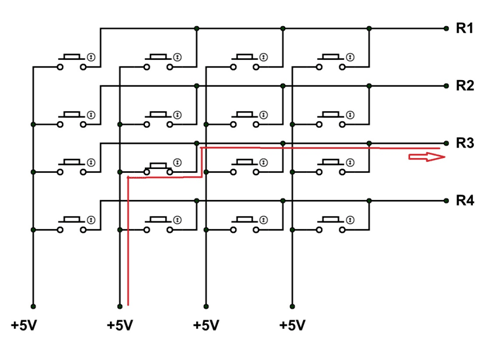
Step2: next set all columns to output and set them at +5v. Next set all rows as input to sense the high logic. Since the key pressed is at 2nd column and 3rd row. The current flows as shown below.
With that current flow a positive voltage of +5v appears at r3 pin. Since all rows are set as inputs, the controller can sense +5v at r3 pin. The controller can be programmed to remember the key being pressed at third row of keypad matrix.
From previous step, we have known the column number of key pressed and now we know row number. With that we can match the key being pressed. We can take the key input provided by this way for 4x4 keypad module.
4x4 keypad module Datasheet
Installing Library
To install the library navigate to the Sketch > Include Library > Manage Libraries… Wait for Library Manager to download libraries index and update list of installed libraries.
Keypad.h : you need to Download and install the keypad library.
LiquidCrystal_I2C.h : you need to Download and install the LiquidCrystal_I2C library.
After installing the required libraries, copy the following code to your Arduino IDE.
Arduino Code
#include <Keypad.h>
#include <EEPROM.h>
#include <LiquidCrystal_I2C.h>
LiquidCrystal_I2C lcd(0x27,16,2); // set the LCD address to 0x27 for a 16 chars and 2 line display
#include <Servo.h>
Servo myservo;
#define LEDindicator A3 //Door Open/close LED indicator
#define BypassSwitch A2 //Push Button for emergency bypass
int pos = 0; // variable to store the servo position
const byte numRows= 4; //number of rows on the keypad
const byte numCols= 4; //number of columns on the keypad
char keymap[numRows][numCols]=
{
{'1', '2', '3', 'A'},
{'4', '5', '6', 'B'},
{'7', '8', '9', 'C'},
{'*', '0', '#', 'D'}
};
char keypressed;
char code[]= {'1','2','3','4'}; //The default password
char code_buff1[sizeof(code)]; //Where the new password is stored
char code_buff2[sizeof(code)]; //Where the new password is stored again
short a=0,i=0,s=0,j=0; //Variables
byte rowPins[numRows] = {8,9,10,11}; //Rows 0 to 3
byte colPins[numCols]= {2,3,4,5}; //Columns 0 to 3
Keypad myKeypad= Keypad(makeKeymap(keymap), rowPins, colPins, numRows, numCols);
void setup()
{
Serial.begin(9600);
lcd.init(); // initialize the lcd
// Print a message to the LCD.
lcd.backlight();
lcd.setCursor(0,0);
lcd.print("ACCESS PROTECTED");
lcd.setCursor(0,1);
lcd.print("ENTER *(PW)0 ");
myservo.attach(7); // attaches the servo on pin 9 to the servo object
pinMode(LEDindicator,OUTPUT);
pinMode(BypassSwitch,INPUT);
for(i=0 ; i<sizeof(code);i++){ // uncomment
EEPROM.get(i, code[i]); // uncomment
}
}
void loop()
{
keypressed = myKeypad.getKey(); //4x4 key to be pressed
if(keypressed == '*'){ // * to open the lock
lcd.clear();
lcd.setCursor(0,0);
lcd.print("ENTER (PW)0 ");
GetCode(); //Getting code
if(a==sizeof(code)) //The size of the code array
OpenDoor();
else{
lcd.clear();
lcd.setCursor(0,0);
lcd.print("WRONG PASSWORD ");
lcd.setCursor(0,1);
lcd.print("TRY AGAIN ");
}
delay(2000);
lcd.clear();
lcd.setCursor(0,0);
lcd.print("ACCESS PROTECTED");
lcd.setCursor(0,1);
lcd.print("ENTER *(PW)0 ");
}
if(keypressed == '#'){ //To change the code it calls the Password Change function
PWChange();
// lcd.clear();
// lcd.print("ENTER PASSWORD ");
}
if(digitalRead(BypassSwitch)==HIGH){ //Opening door
digitalWrite(LEDindicator,HIGH);
lcd.setCursor(0,0);
lcd.print("BYPASS ACTIVATED");
lcd.setCursor(0,1);
lcd.print("DOOR OPENING....");
for (pos = 90; pos <= 180; pos += 1) { // goes from 0 degrees to 180 degrees in steps of 1 degree
myservo.write(pos); // tell servo to go to position in variable 'pos'
delay(15); // waits 15 ms for the servo to reach the position
}
delay(3000);
for (pos = 180; pos >= 90; pos -= 1) { // goes from 180 degrees to 0 degrees
myservo.write(pos); // tell servo to go to position in variable 'pos'
delay(15); // waits 15 ms for the servo to reach the position
}
// delay(3000); //Opens for 3s you can change
digitalWrite(LEDindicator,LOW);
lcd.clear();
lcd.setCursor(0,0);
lcd.print("ACCESS PROTECTED");
lcd.setCursor(0,1);
lcd.print("ENTER *(PW)0 ");
}
}
void GetCode(){ //Getting code sequence
i=0; //All variables set to 0
a=0;
j=0;
while(keypressed != '0'){ //The user press 0 to confirm the code otherwise he can keep typing
keypressed = myKeypad.getKey();
if(keypressed != NO_KEY && keypressed != '0' ){ //If the char typed isn't 0 and neither "nothing"
lcd.setCursor(j,1); //This to write "*" on the LCD whenever a key is pressed it's position is controlled by j
lcd.print("*");
j++;
if(keypressed == code[i]&& i<sizeof(code)){ //if the char typed is correct a and i increments to verify the next caracter
a++; //Now I think maybe I should have use only a or i ... too lazy to test it -_-'
i++;
}
else
a--; //if the character typed is wrong a decrements and cannot equal the size of code []
}
}
keypressed = NO_KEY;
}
void PWChange(){ //Change code sequence
lcd.clear();
lcd.print("PASSWORD CHANGE ");
delay(1000);
lcd.clear();
lcd.print("ENTER (old PW)0 ");
GetCode(); //verify the old code first so you can change it
if(a==sizeof(code)){ //again verifying the a value
lcd.clear();
lcd.print("PASSWORD CHANGE ");
PWChangeCode(); //Get the new code
PWChangeconfirm(); //Get the new code again to confirm it
s=0;
for(i=0 ; i<sizeof(code) ; i++){ //Compare codes in array 1 and array 2 from two previous functions
if(code_buff1[i]==code_buff2[i])
s++; //again this how we verifiy, increment s whenever codes are matching
}
if(s==sizeof(code)){ //Correct is always the size of the array
for(i=0 ; i<sizeof(code) ; i++){
code[i]=code_buff2[i]; //the code array now receives the new code
EEPROM.put(i, code[i]); //And stores it in the EEPROM
//Serial.print(EEPROM.get(i, code[i])); //password
}
lcd.clear();
lcd.print("PASSWORD CHANGED");
delay(2000);
}
else{ //In case the new codes aren't matching
lcd.clear();
lcd.print("NEW PASSWORD ARE");
lcd.setCursor(0,1);
lcd.print("NOT MATCHING !! ");
delay(2000);
lcd.setCursor(0,0);
lcd.print("WRONG PASSWORD ");
lcd.setCursor(0,1);
lcd.print("TRY AGAIN ");
}
}
delay(2000);
lcd.clear();
lcd.setCursor(0,0);
lcd.print("ACCESS PROTECTED");
lcd.setCursor(0,1);
lcd.print("ENTER *(PW)0 ");
}
void PWChangeCode(){
i=0;
j=0;
lcd.clear();
lcd.print("CHANGE TO NEW PW");
lcd.setCursor(0,1);
lcd.print("ENTER (new PW)0 ");
delay(2000);
lcd.clear();
lcd.setCursor(0,1);
lcd.print("ENTER (new PW)0 ");
while(keypressed != '0'){ //0 to confirm and quits the loop
keypressed = myKeypad.getKey();
if(keypressed != NO_KEY && keypressed != '0' ){
lcd.setCursor(j,0);
lcd.print("*"); //On the new code you can show * as I did or change it to keypressed to show the keys
code_buff1[i]=keypressed; //Store caracters in the array
i++;
j++;
}
}
keypressed = NO_KEY;
}
void PWChangeconfirm(){ //This is exactly like the ChangeCode function but this time the code is stored in another array
i=0;
j=0;
lcd.clear();
lcd.print("CONFIRM PASSWORD");
lcd.setCursor(0,1);
lcd.print("ENTER (new PW)0 ");
delay(3000);
lcd.clear();
lcd.setCursor(0,1);
lcd.print("ENTER (new PW)0 ");
while(keypressed != '0'){
keypressed = myKeypad.getKey();
if(keypressed != NO_KEY && keypressed != '0' ){
lcd.setCursor(j,0);
lcd.print("*");
code_buff2[i]=keypressed;
i++;
j++;
}
}
keypressed = NO_KEY;
}
void OpenDoor(){ //Door opening function open for 3s
lcd.clear();
lcd.setCursor(0,0);
lcd.print("ACCESS GRANTED ");
lcd.setCursor(0,1);
lcd.print("DOOR OPENING....");
digitalWrite(LEDindicator,HIGH);
for (pos = 90; pos <= 180; pos += 1) { // goes from 0 degrees to 180 degrees
// in steps of 1 degree
myservo.write(pos); // tell servo to go to position in variable 'pos'
delay(15); // waits 15 ms for the servo to reach the position
}
delay(3000);
for (pos = 180; pos >= 90; pos -= 1) { // goes from 180 degrees to 0 degrees
myservo.write(pos); // tell servo to go to position in variable 'pos'
delay(15); // waits 15 ms for the servo to reach the position
}
digitalWrite(LEDindicator,LOW);
}
Demo Video
Subscribe and Download code in case of forget password to erase EEPROM values in address (without EEPROM).
I recently had security password-protected doors installed by Locksmith Gastonia NC company, and I couldn't be happier with the results. The level of security these doors provide is unmatched, giving me peace of mind knowing that my home is well-protected.