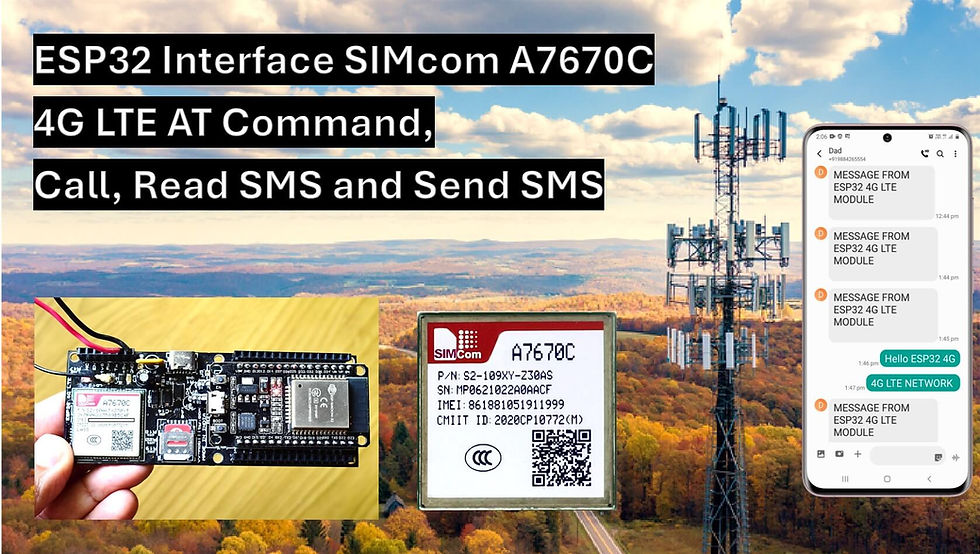
In this tutorial, you'll learn how to use Simcom 7670C 4G LTE Module, An Integrating the SIM7670C 4G LTE module with an ESP32 and using AT commands to make calls and send / receive SMS is a powerful way to leverage cellular connectivity in your projects. Here’s a step-by-step guide to get you started with this:.
Circuit Diagram:
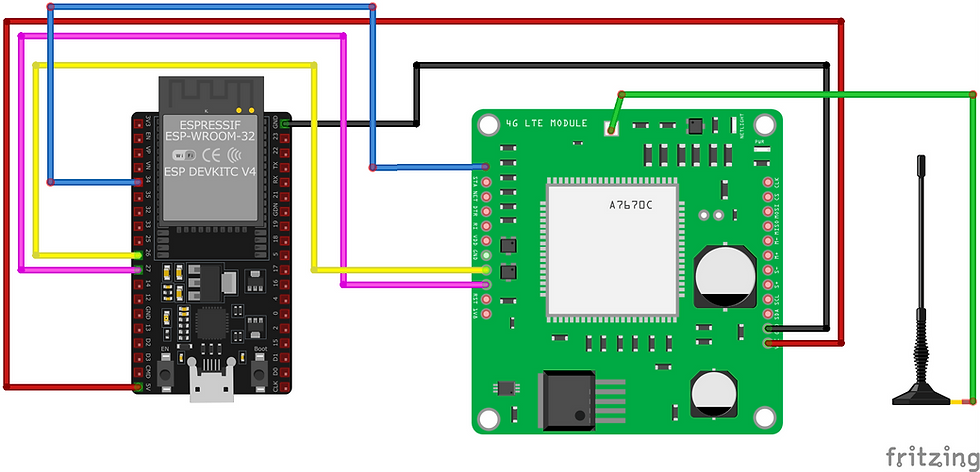
Components Required:
ESP32 Development Board
SIM7670C 4G LTE Module
SIM Card with Data Plan
Connecting Wires
Power Supply (if needed)
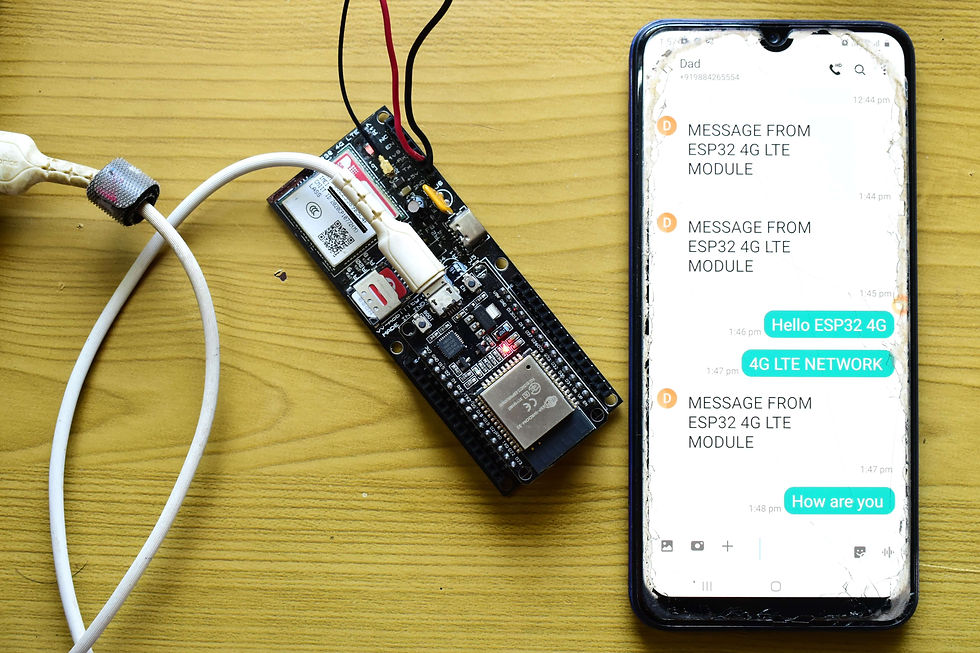
SIMCom7670C
The SIM A7670C is a compact and robust development board that integrates the SIMCom A7670C module, a high-performance 4G LTE Cat-1 module with fallback to 2G networks. This board is designed to provide reliable and efficient communication solutions for a wide range of IoT applications, including smart meters, asset tracking, and remote monitoring systems.
The module support GSM, LTE-TDD and LTE-FDD. Users can choose the module according to the wireless network configuration. The supported radio frequency bands are described in the data sheet.
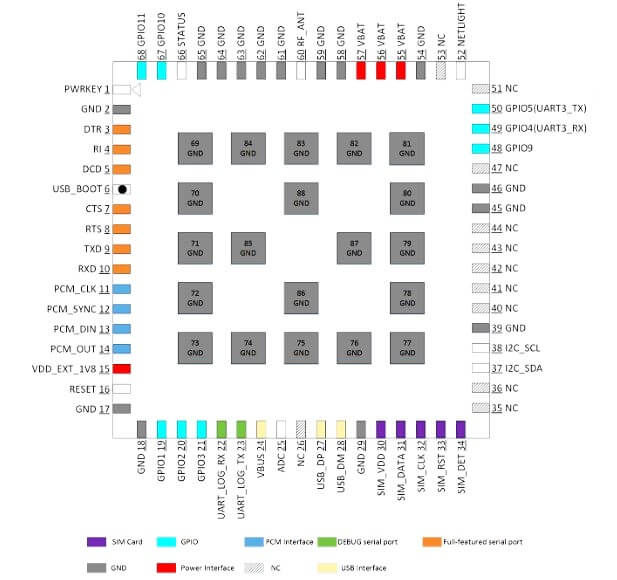
Pin Assignment overview
All functions of the module will be provided through 88 pads that will be connected to the customers’ platform. The following Figure is a high-level view of the pin assignment of the module.
Wiring the SIM7670C with ESP32
Connect the SIM7670C to ESP32:
SIM7670C TX -> ESP32 RX (e.g., GPIO 27)
SIM7670C RX -> ESP32 TX (e.g., GPIO 24)
SIM7670C PowerKey -> ESP32 TX (e.g., GPIO 4)
SIM7670C GND -> ESP32 GND
SIM7670C VCC -> ESP32 3.3V or External 4G Power Supply (Check the module’s voltage requirements. Some modules need 4V to 5V, so use an appropriate power supply if needed.)
Ensure proper power supply to avoid potential damage to the module or unstable operation.
To start developing with the SIM A7670C, you’ll need a few essential components and tools:
SIM A7670C Development Board: The main development board with the SIMCom A7670C module.
Microcontroller or Development Platform: Such as Arduino, Raspberry Pi, or any other platform with UART or USB interface.
SIM Card: A SIM card with an active data plan to enable network connectivity.
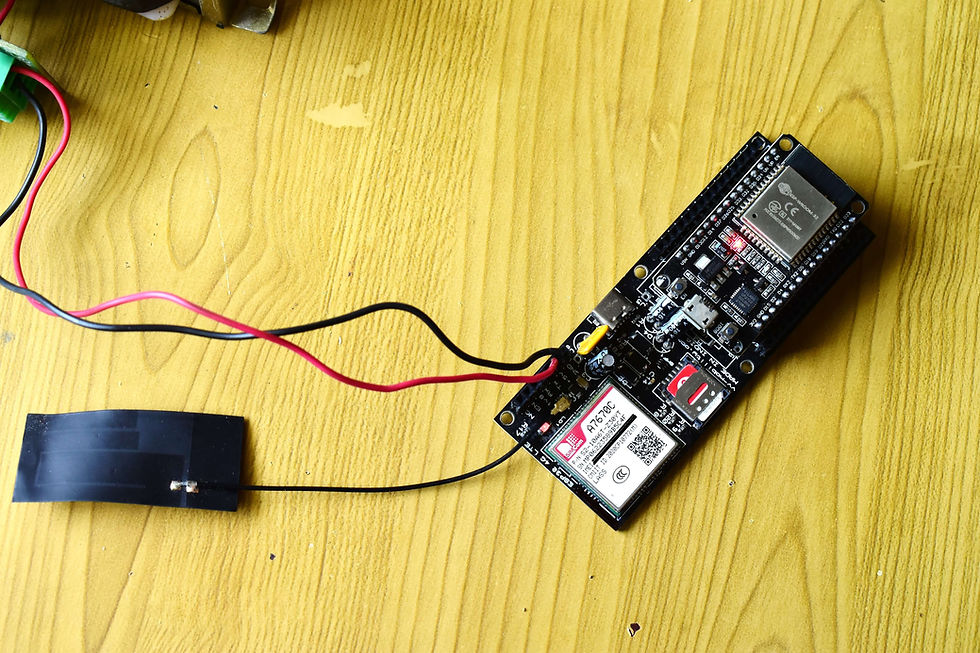
Setup Arduino IDE for ESP32
Install the ESP32 Board Package:
Open Arduino IDE.
Go to File > Preferences.
Add the following URL to the "Additional Board Manager URLs" field: https://dl.espressif.com/dl/package_esp32_index.json.
Go to Tools > Board > Boards Manager, search for ESP32, and install it.
Install the Required Libraries:
Generally, no additional libraries are required for basic AT command communication. However, if you need specific libraries for advanced features, you can install them via the Library Manager.
Tiny GSM Arduino library for GSM modules,
After installed the libraries, restart your Arduino IDE.
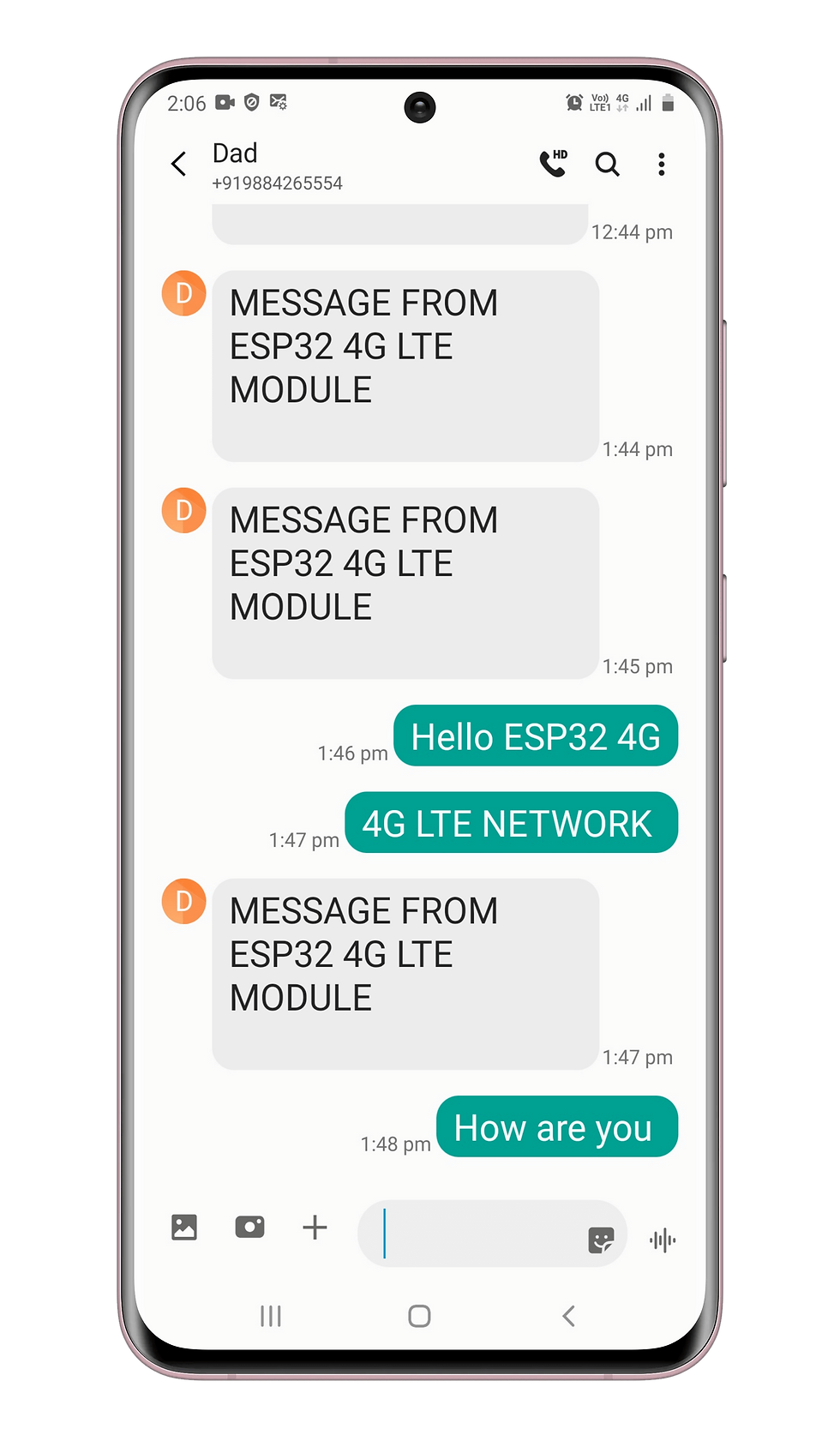
AT COMMAND Arduino Code
#define TINY_GSM_MODEM_SIM7600 // SIM7600 AT instruction is compatible with A7670
#define SerialAT Serial1
#define SerialMon Serial
#define TINY_GSM_USE_GPRS true
#include <TinyGsmClient.h>
#define RXD2 27
#define TXD2 26
#define powerPin 4
char a, b;
const char apn[] = ""; //APN automatically detects for 4G SIM, NO NEED TO ENTER, KEEP IT BLANK
#ifdef DUMP_AT_COMMANDS
#include <StreamDebugger.h>
StreamDebugger debugger(SerialAT, SerialMon);
TinyGsm modem(debugger);
TinyGsm modem(SerialAT);
TinyGsmClient client(modem);
void setup()
{
Serial.begin(115200);
pinMode(powerPin, OUTPUT);
digitalWrite(powerPin, LOW);
delay(100);
digitalWrite(powerPin, HIGH);
delay(1000);
digitalWrite(powerPin, LOW);
Serial.println("\nconfiguring A7670C Module. Kindly wait");
delay(10000);
SerialAT.begin(115200, SERIAL_8N1, RXD2, TXD2);
// Restart takes quite some time
// To skip it, call init() instead of restart()
DBG("Initializing modem...");
if (!modem.init()) {
DBG("Failed to restart modem, delaying 10s and retrying");
return;
}
// Restart takes quite some time
// To skip it, call init() instead of restart()
DBG("Initializing modem...");
if (!modem.restart()) {
DBG("Failed to restart modem, delaying 10s and retrying");
return;
}
String name = modem.getModemName();
DBG("Modem Name:", name);
String modemInfo = modem.getModemInfo();
DBG("Modem Info:", modemInfo);
Serial.println("Waiting for network...");
if (!modem.waitForNetwork()) {
Serial.println(" fail");
delay(10000);
return;
}
Serial.println(" success");
if (modem.isNetworkConnected()) {
Serial.println("Network connected");
}
// GPRS connection parameters are usually set after network registration
Serial.print(F("Connecting to "));
Serial.print(apn);
if (!modem.gprsConnect(apn)) {
Serial.println(" fail");
delay(10000);
return;
}
Serial.println(" success");
if (modem.isGprsConnected()) {
Serial.println("LTE module connected");
}
Serial.println("Enter Standard AT commands like AT, AT+CPIN?, AT+CCLK?, etc.");
Serial.println("SELECT SERIAL PORT MONITOR AS \"BOTH NL & CR\" TO VIEW COMMAND RESPONSE CORRECTLY IF YOU ARE USING ARDUINO IDE");
Serial.println("Refer A7600 series datasheet for entire list of commands");
Serial.println("Understand the AT Commands properly");
Serial.println("Incorrect AT commands can corrupt the 4G module memory!!!");
// MQTT Broker setup
//mqtt.setServer(broker, 1883);
//mqtt.setCallback(callback);
}
void loop()
{
if (Serial.available() > 0) // read AT commands from user Serial port and send to the Module
{
a = Serial.read();
SerialAT.write(a);
}
if (SerialAT.available() > 0) //read Response commands from module and send to user Serial Port
{
b = SerialAT.read();
Serial.write(b);
}
}
After a successful upload, open the Serial Monitor at a baud rate of 115200. Press the “EN/RST” button on the ESP32 board.
Basic AT Command Testing
Upload Basic Code to ESP32: Open the Arduino IDE and load the following sketch to test AT commands via the Serial Monitor.
Upload this code to the ESP32.
Open the Serial Monitor (set to 115200 baud rate) to interact with the SIM7670C module.
Test Basic AT Commands: In the Serial Monitor, type AT and press Enter. You should receive an OK response if the module is correctly connected.
A7670 Module Connect
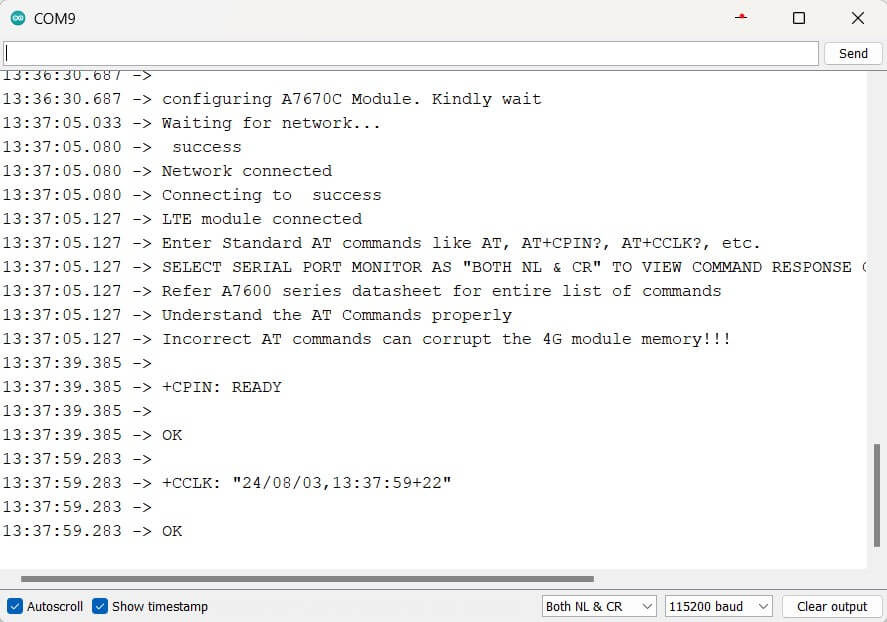
AT Command Manual : https://www.ktron.in/wp-content/uploads/2023/03/A76XX-Series_AT_Command_Manual_V1.06-4.pdf
AT COMMAND Call/ Send SMS Arduino Code
#define RXD2 27
#define TXD2 26
#define powerPin 4
int rx = -1;
#define SerialAT Serial1
String rxString;
int _timeout;
String _buffer;
String number = "+919500950137"; // send/call replace mobile number enter here
void setup() {
pinMode(powerPin, OUTPUT);
digitalWrite(powerPin, LOW);
Serial.begin(115200);
delay(100);
SerialAT.begin(115200, SERIAL_8N1, RXD2, TXD2);
delay(10000);
Serial.println("Modem Reset, please wait");
SerialAT.println("AT+CRESET");
delay(1000);
SerialAT.println("AT+CRESET");
delay(20000); // WAITING FOR SOME TIME TO CONFIGURE MODEM
SerialAT.flush();
Serial.println("Echo Off");
SerialAT.println("ATE0"); //120s
delay(1000);
SerialAT.println("ATE0"); //120s
rxString = SerialAT.readString();
Serial.print("Got: ");
Serial.println(rxString);
rx = rxString.indexOf("OK");
if (rx != -1)
Serial.println("Modem Ready");
delay(1000);
Serial.println("SIM card check");
SerialAT.println("AT+CPIN?"); //9s
rxString = SerialAT.readString();
Serial.print("Got: ");
Serial.println(rxString);
rx = rxString.indexOf("+CPIN: READY");
if (rx != -1)
Serial.println("SIM Card Ready");
delay(1000);
Serial.println("Type s to send an SMS, r to receive an SMS, and c to make a call");
}
void loop() {
if (Serial.available() > 0)
switch (Serial.read())
{
case 's':
SendMessage(); //YOU CAN SEND MESSAGE FROM SIM TO THE MENTIONED PHONE NUMBER
break;
case 'r':
RecieveMessage(); // RECEIVE MESSAGE FROM THE MENTIONED PHONE NUMBER TO SIM
break;
case 'c':
callNumber(); // CALL
break;
}
if (SerialAT.available() > 0)
Serial.write(SerialAT.read());
}
void SendMessage()
{
//Serial.println ("Sending Message");
SerialAT.println("AT+CMGF=1"); //Sets the GSM Module in Text Mode
delay(1000);
//Serial.println ("Set SMS Number");
SerialAT.println("AT+CMGS=\"" + number + "\"\r"); //Mobile phone number to send message
delay(1000);
String SMS = "MESSAGE FROM ESP32 4G LTE MODULE"; //replace message here
SerialAT.println(SMS);
delay(100);
SerialAT.println((char)26);// ASCII code of CTRL+Z
delay(1000);
_buffer = _readSerial();
}
void RecieveMessage()
{
Serial.println ("AT7670C Read an SMS");
delay (1000);
SerialAT.println("AT+CNMI=2,2,0,0,0"); // AT Command to receive a live SMS
delay(1000);
Serial.write ("Unread Message done");
}
String _readSerial() {
_timeout = 0;
while (!SerialAT.available() && _timeout < 12000 )
{
delay(13);
_timeout++;
}
if (SerialAT.available()) {
return SerialAT.readString();
}
}
void callNumber() {
SerialAT.print (F("ATD"));
SerialAT.print (number);
SerialAT.print (F(";\r\n"));
_buffer = _readSerial();
Serial.println(_buffer);
}
After a successful upload, open the Serial Monitor at a baud rate of 115200. Press the “EN/RST” button on the ESP32 board.
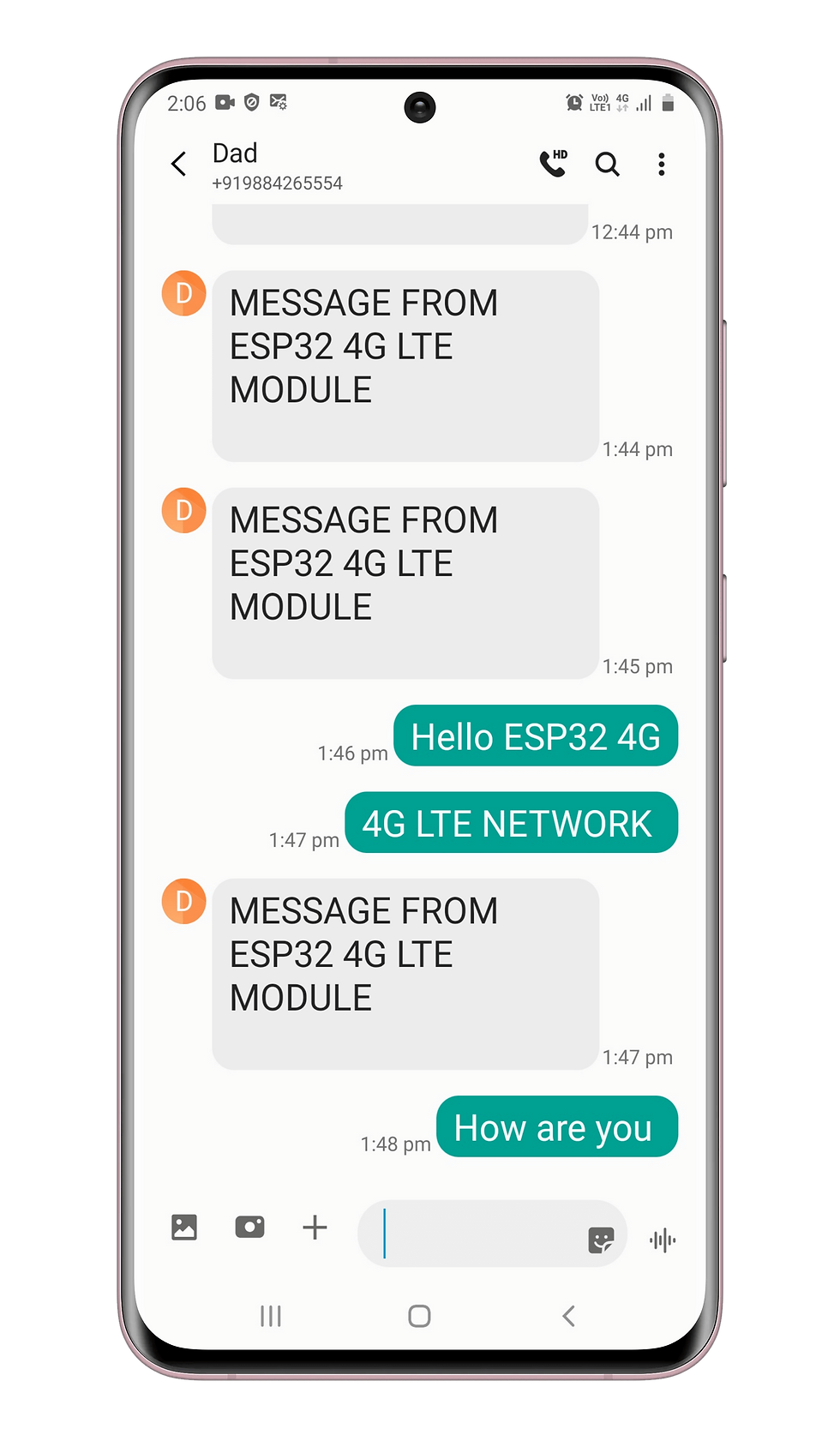
Making Calls and Sending / Receive SMS
Here’s how you can extend the functionality to make calls and send SMS.
Code to Make Calls and Send / Receive SMS:
Replace "+1234567890" with the phone number you want to call or send SMS
Upload the Code and Test:
Upload the sketch to the ESP32.
Open the Serial Monitor to observe the AT commands being sent and the responses from the module.
Command "s" - send SMS
Command "r" - read SMS
Command "c" - call
Refer below for serial monitor output:
A7670 Modem Check:
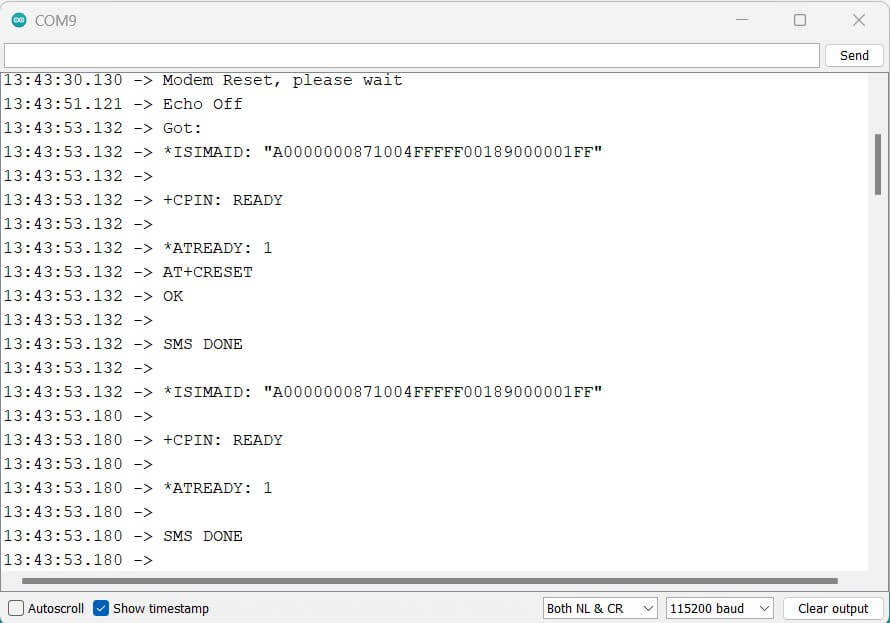
A7670 Modem Ready
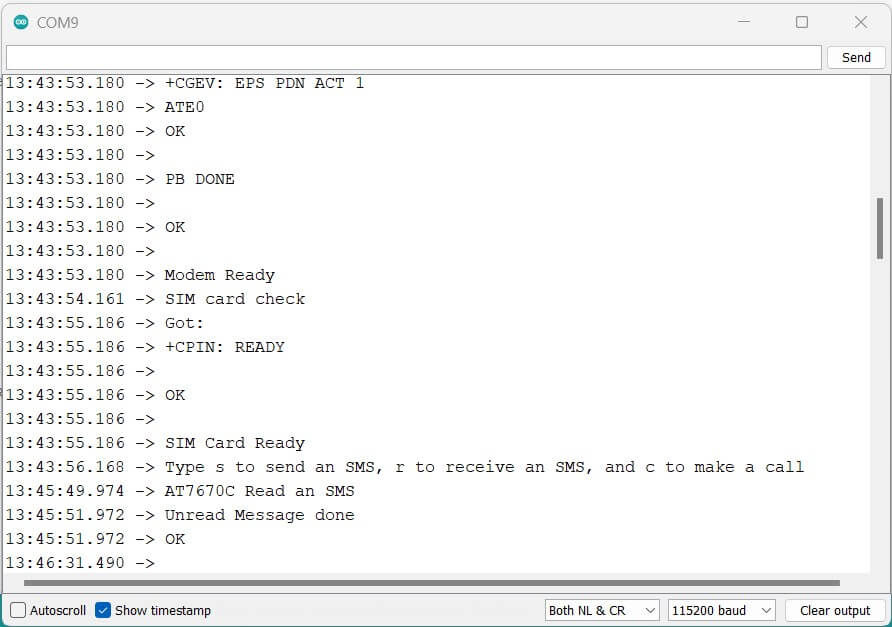
AT Command : Read/Send SMS
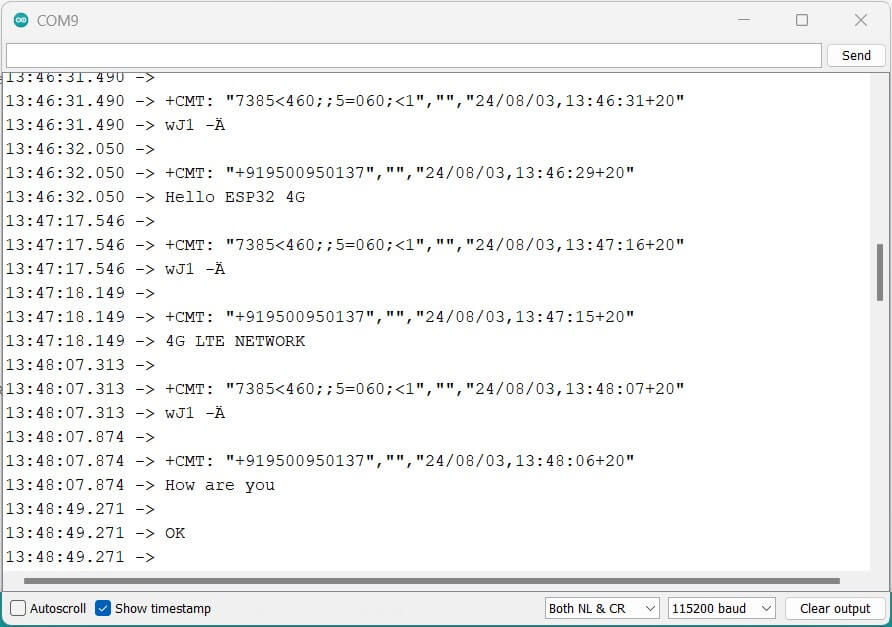
AT Command : To Call
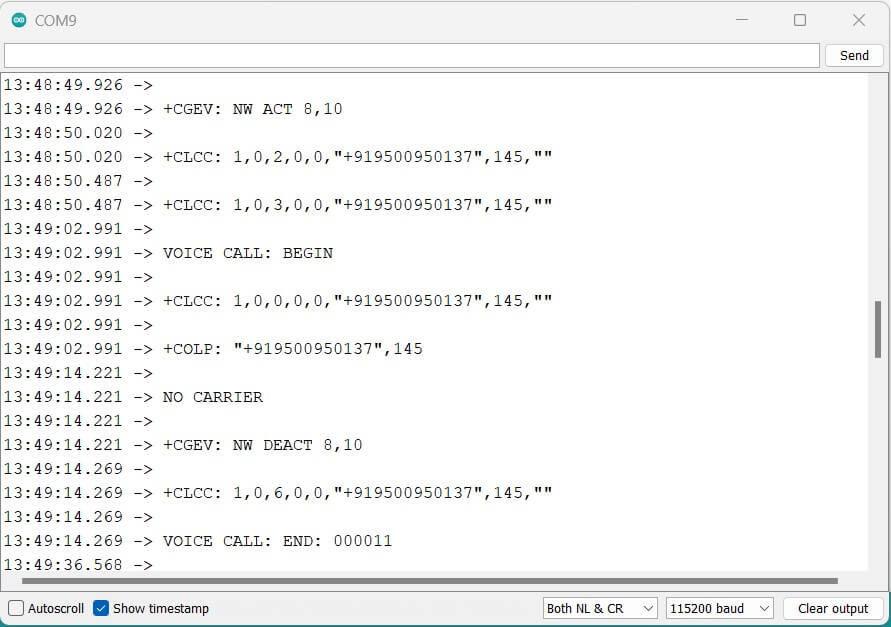
AT Command : Receive Call

Mobile Log:
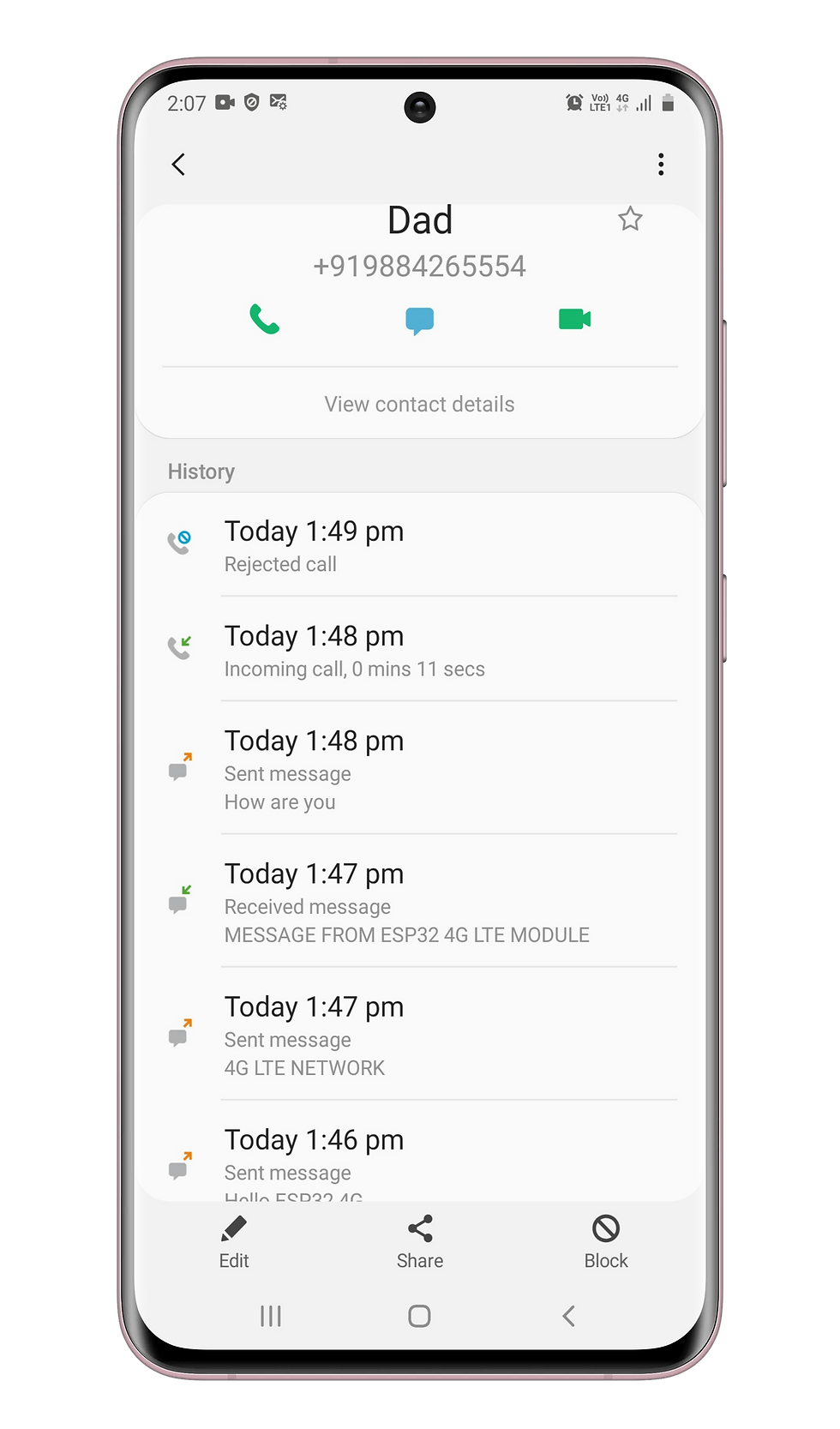
Network Timeout:

Demo:
Troubleshooting
No Response: Check wiring connections and ensure the module is powered correctly.
Command Errors: Make sure AT commands are formatted correctly and the module supports them.
Module Not Found: Verify the baud rate and serial port settings match those of the SIM7670C.
This guide should give you a good starting point for using the SIM7670C with the ESP32 and testing various AT commands. Let me know if you have any specific questions or run into issues!
Comments