ESP32 3.5inch TFT Touch display ILI9488 with LVGL UI Part5
- Ramesh G
- Oct 7, 2024
- 6 min read
Updated: Oct 20, 2024
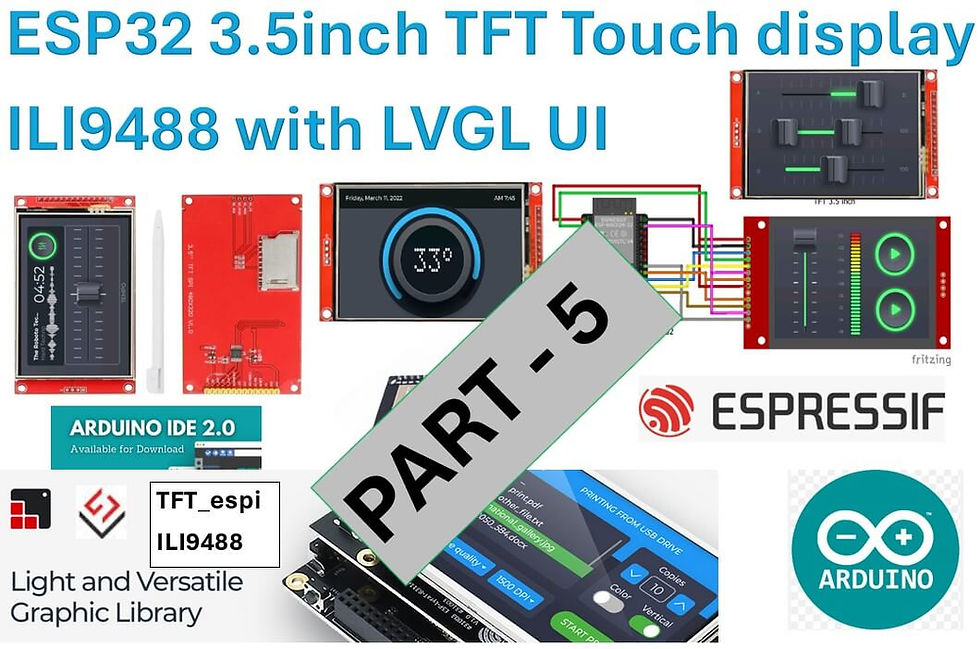
Here to learn how to make a ESP32 board based 3.5inch touch display ILI9488 using the LVGL (Light and Versatile Graphics Library) and Bodmer's TFT_eSPI arduino Library. The LVGL is a popular free and open-source embedded graphics library to create UIs for arduino.
In this Setting up LVGL (Light and Versatile Graphics Library) on an ESP32 with a TFT LCD touchscreen display ILI9488 is a great way to create interactive user interfaces for your projects. Here’s a step-by-step guide to help you get started.

Demo:
Components Required
ESP-32 Module (38Pin)
3.5 inch TFT LCD Display Module SPI Interface 320x480 with Touch Screen
Jumper Wires
Circuit Diagram

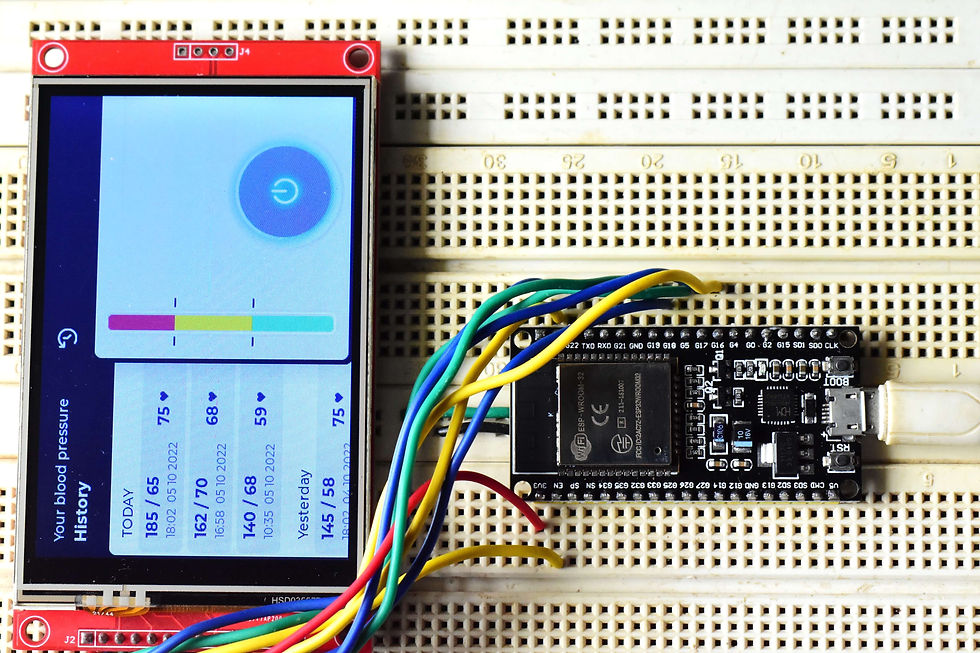
TFT refer blog:
In the VCC pin, you can either use 5V or 3.3V depending upon if your J1 connection is open or closed,
J1 = open=5V; J1=close=3.3V.
LVGL refer:
Hardware Requirements
ESP32 Board: Any variant should work, but make sure it has enough GPIO pins.
TFT LCD Touchscreen: Common models include ILI9488
Connecting Wires: Jumper wires for connections.
Breadboard (optional): For easier connections.
Software Requirements
Arduino IDE: Make sure you have the latest version.
Select “File>Preferences>settings>Additional Boards Manager URLs” to fill the link.
Here are the steps to install the ESP32 board in Arduino IDE:
Open the Arduino IDE
Select File and then Preferences
Find the Additional Board Manager URLs field and activate the text box
Add the URL to the field
Click OK to save the changes
https://raw.githubusercontent.com/espressif/arduino-esp32/gh-pages/package_esp32_dev_index.json,
https://arduino.esp8266.com/stable/package_esp8266com_index.json,
https://espressif.github.io/arduino-esp32/package_esp32_index.json
LVGL Library: Available through the Library Manager in the Arduino IDE.
Touch Driver Library: Depending on your touchscreen (e.g., TFT_eSPI)
Configuring LVGL
LVGL Configuration: You may need to configure LVGL for your specific display and touch screen. Create a lv_conf.h file or modify the existing one in the LVGL library folder:
Set LVGL_DISPLAY_HOR_RES and LVGL_DISPLAY_VER_RES to match your display's resolution.
Configure the display and touch input settings based on your library (e.g., TFT_eSPI settings).
TFT_eSPI Configuration:
A feature rich Arduino IDE compatible graphics and fonts library for 32-bit processors. The library is targeted at 32-bit processors, it has been performance optimised for RP2040, STM32, ESP8266 and ESP32 types, other 32-bit processors may be used but will use the slower generic Arduino interface calls. The library can be loaded using the Arduino IDE's Library Manager. Direct Memory Access (DMA) can be used with the ESP32, RP2040 and STM32 processors with SPI interface displays to improve rendering performance. DMA with a parallel interface (8 and 16-bit) is only supported with the RP2040.
Go to the TFT_eSPI library folder and open the User_Setup.h file to configure the pins according to your wiring.
Wiring the TFT Display
TFT Pin | ESP32 Pin |
VCC | 3.3V |
GND | GND |
CS | GPIO 5 |
RESET | GPIO 2 |
DC/RS | GPIO 17 |
SDI(MOSI) | GPIO 23 |
SCK | GPIO 18 |
LED | 3.3V (or PWM pin for brightness) |
Wiring the Touchscreen (e.g., TFT_espi)
Touch Pin | ESP32 Pin |
T_CS | GPIO 16 |
T_IRQ | GPIO 21 |
T_DOUT | GPIO 19 |
T_DIN | GPIO 23 |
T_CLK | GPIO 18 |
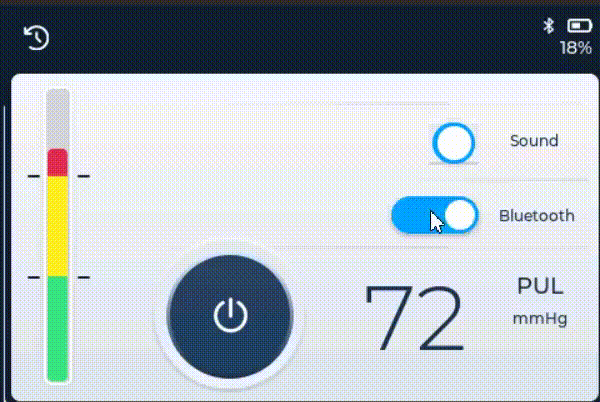
Sample Code
Here’s a basic example to initialize the display and create a simple button using LVGL:
Example 5.
###########################################################################
//Arduino-TFT_eSPI board-template main routine. There's a TFT_eSPI create+flush driver already in LVGL-9.1 but we create our own here for more control (like e.g. 16-bit color swap).
#include "FS.h"
#include <SPI.h>
#include <lvgl.h>
#include <TFT_eSPI.h>
#include <ui.h>
/*Don't forget to set Sketchbook location in File/Preferences to the path of your UI project (the parent foder of this INO file)*/
/*Change to your screen resolution*/
static const uint16_t screenWidth = 480;
static const uint16_t screenHeight = 320;
enum { SCREENBUFFER_SIZE_PIXELS = screenWidth * screenHeight / 10 };
static lv_color_t buf [SCREENBUFFER_SIZE_PIXELS];
TFT_eSPI tft = TFT_eSPI( screenWidth, screenHeight ); /* TFT instance */
#define CALIBRATION_FILE "/calibrationData"
#if LV_USE_LOG != 0
/* Serial debugging */
void my_print(const char * buf)
{
Serial.printf(buf);
Serial.flush();
}
/* Display flushing */
void my_disp_flush (lv_display_t disp, const lv_area_t area, uint8_t *pixelmap)
{
uint32_t w = ( area->x2 - area->x1 + 1 );
uint32_t h = ( area->y2 - area->y1 + 1 );
if (LV_COLOR_16_SWAP) {
size_t len = lv_area_get_size( area );
lv_draw_sw_rgb565_swap( pixelmap, len );
}
tft.startWrite();
tft.setAddrWindow( area->x1, area->y1, w, h );
tft.pushColors( (uint16_t*) pixelmap, w * h, true );
tft.endWrite();
lv_disp_flush_ready( disp );
}
/*Read the touchpad*/
void my_touchpad_read (lv_indev_t indev_driver, lv_indev_data_t data)
{
uint16_t touchX = 0, touchY = 0;
bool touched = tft.getTouch( &touchX, &touchY, 600 );
if (!touched)
{
data->state = LV_INDEV_STATE_REL;
}
else
{
data->state = LV_INDEV_STATE_PR;
/*Set the coordinates*/
data->point.x = touchX;
data->point.y = touchY;
Serial.print( "Data x " );
Serial.println( touchX );
Serial.print( "Data y " );
Serial.println( touchY );
}
}
/*Set tick routine needed for LVGL internal timings*/
static uint32_t my_tick_get_cb (void) { return millis(); }
void setup ()
{
uint16_t calibrationData[5];
uint8_t calDataOK = 0;
Serial.begin( 115200 ); /* prepare for possible serial debug */
String LVGL_Arduino = "Hello Arduino! ";
LVGL_Arduino += String('V') + lv_version_major() + "." + lv_version_minor() + "." + lv_version_patch();
Serial.println( LVGL_Arduino );
Serial.println( "I am LVGL_Arduino" );
lv_init();
#if LV_USE_LOG != 0
lv_log_register_print_cb( my_print ); /* register print function for debugging */
tft.begin(); /* TFT init */
tft.setRotation( 3 ); /* Landscape orientation, flipped */
static lv_disp_t* disp;
disp = lv_display_create( screenWidth, screenHeight );
lv_display_set_buffers( disp, buf, NULL, SCREENBUFFER_SIZE_PIXELS * sizeof(lv_color_t), LV_DISPLAY_RENDER_MODE_PARTIAL );
lv_display_set_flush_cb( disp, my_disp_flush );
static lv_indev_t* indev;
indev = lv_indev_create();
lv_indev_set_type( indev, LV_INDEV_TYPE_POINTER );
lv_indev_set_read_cb( indev, my_touchpad_read );
lv_tick_set_cb( my_tick_get_cb );
ui_init();
Serial.println( "Setup done" );
// check file system
if (!SPIFFS.begin()) {
Serial.println("formatting file system");
SPIFFS.format();
SPIFFS.begin();
}
// check if calibration file exists
if (SPIFFS.exists(CALIBRATION_FILE)) {
if (f) {
if (f.readBytes((char *)calibrationData, 14) == 14)
calDataOK = 1;
f.close();
}
}
if (calDataOK) {
// calibration data valid
tft.setTouch(calibrationData);
} else {
// data not valid. recalibrate
tft.calibrateTouch(calibrationData, TFT_WHITE, TFT_RED, 15);
// store data
if (f) {
f.write((const unsigned char *)calibrationData, 14);
f.close();
}
}
}
void loop ()
{
lv_timer_handler(); /* let the GUI do its work */
delay(5);
}
############################################################################
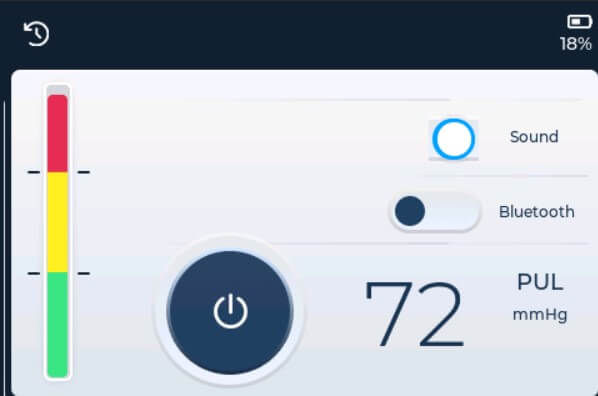
Final Steps
Upload the code to your ESP32 using the Arduino IDE.
Open the Serial Monitor to check for any debug messages.
Interact with the touchscreen to see your UI in action!
Tips
Adjust the pin assignments based on your wiring.
Explore LVGL's extensive documentation for more UI elements and functionalities.
Use LVGL's built-in tools to design and customize your interfaces.
This should give you a solid start on using LVGL with an ESP32 and a TFT LCD touchscreen. Happy coding!
Comments