Creating a demo GUI for an eBike using an ESP32 and a 3.5-inch TFT display can be an exciting project! Below is a high-level overview of how to set up your project, including the necessary components, libraries, and a basic example of how to structure your code.
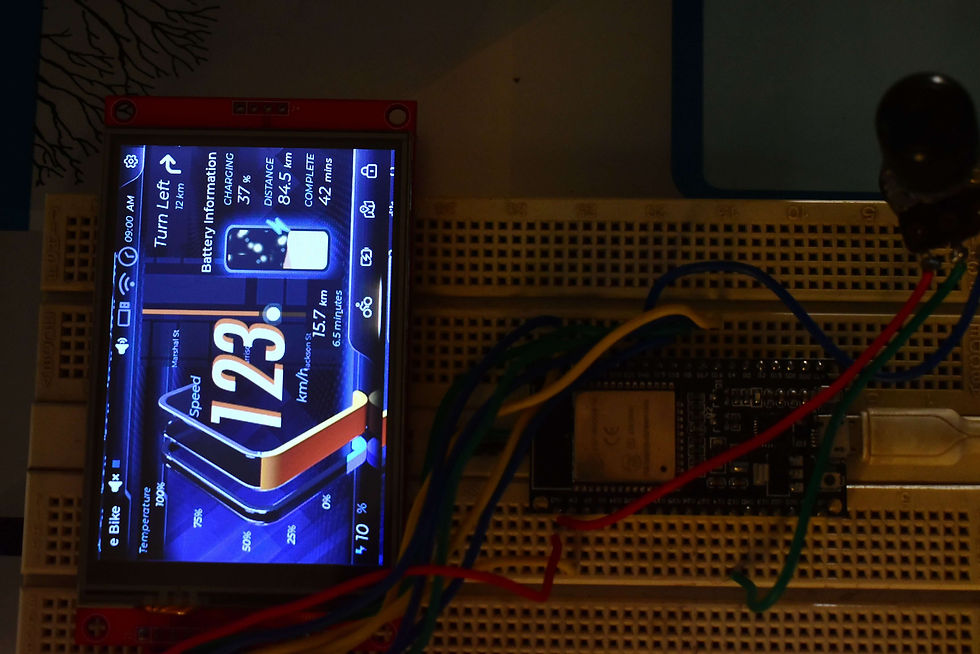
Components Required
ESP-32 Module (38Pin)
3.5 inch TFT ILI9488 SPI Interface Module 480x320 with Touch Screen Display
10k Potentiometer -2nos
Jumper Wires
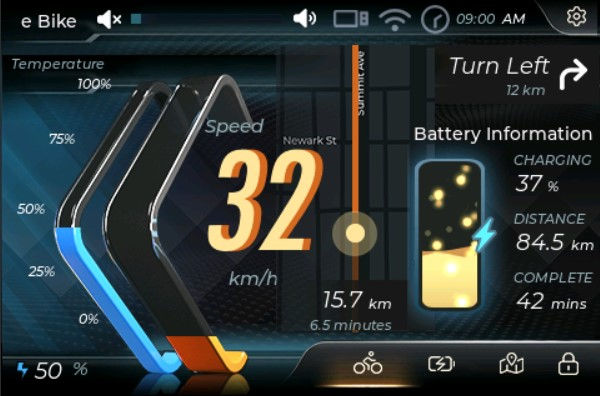
Circuit Diagram
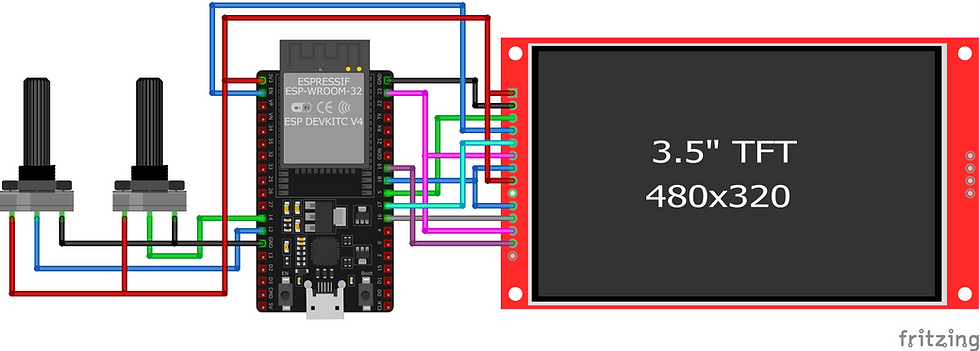
3.5inch TFT display
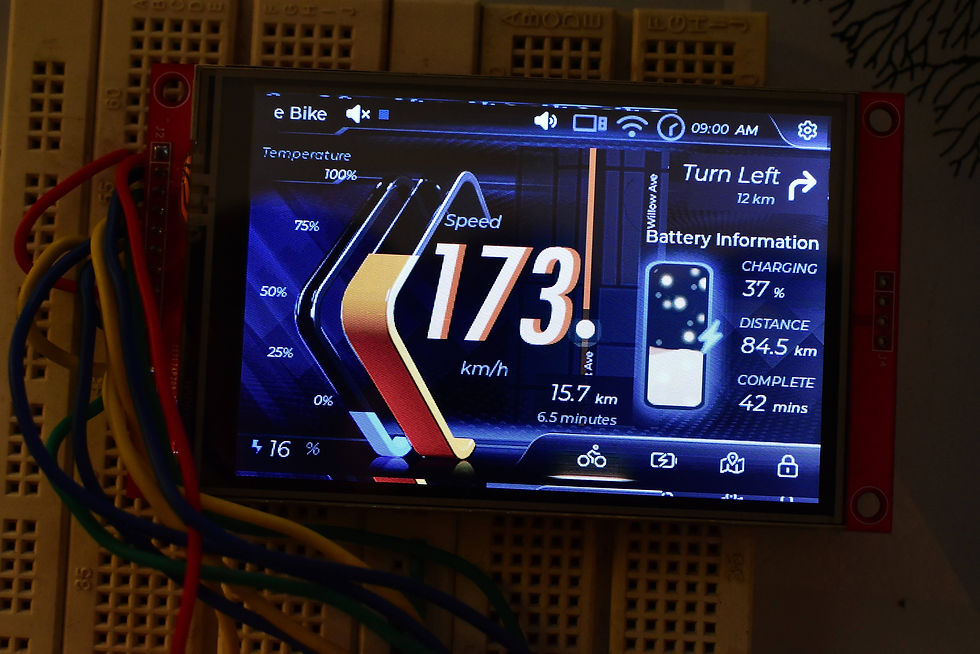
TFT refer blog:
In the VCC pin, you can either use 5V or 3.3V depending upon if your J1 connection is open or closed,
J1 = open=5V; J1=close=3.3V.
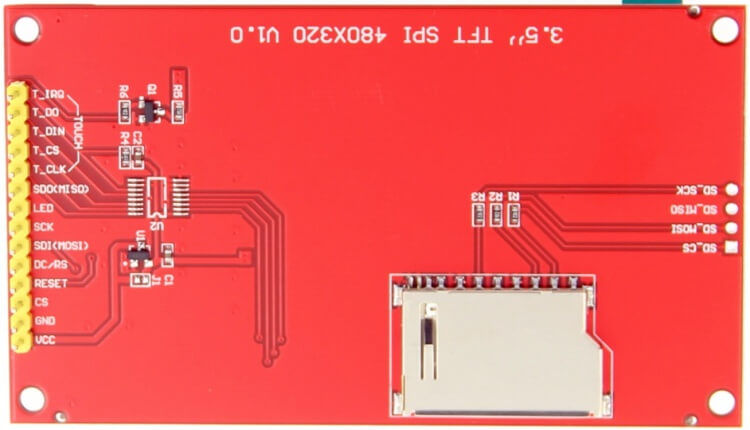
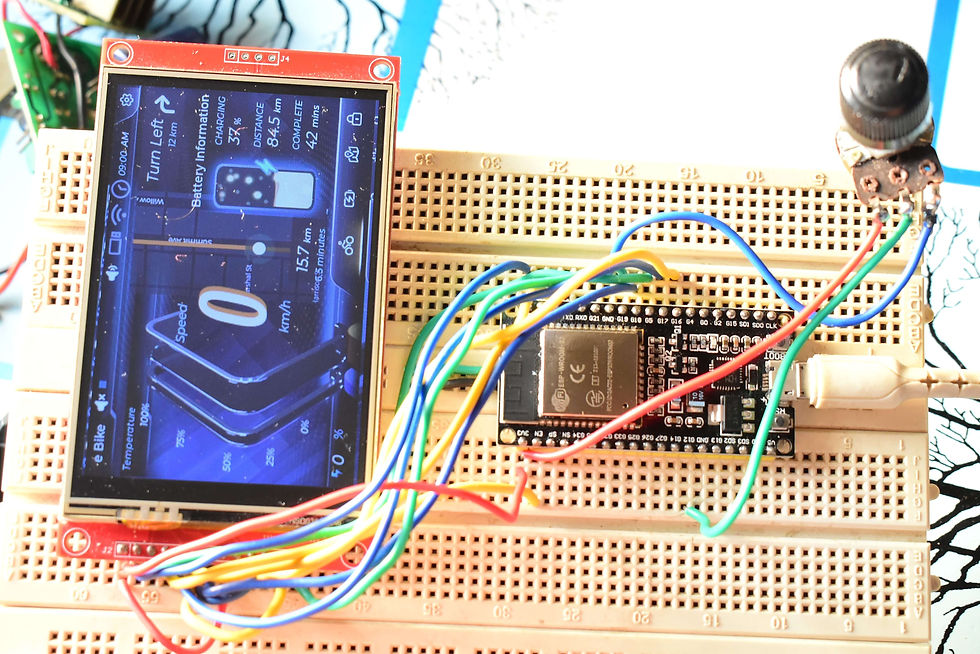
LVGL refer:
Hardware Requirements
ESP32 Board: Any variant should work, but make sure it has enough GPIO pins.
TFT LCD Touchscreen: Common models include ILI9488
Connecting Wires: Jumper wires for connections.
Breadboard (optional): For easier connections.
Software Requirements
Arduino IDE: Make sure you have the latest version.
Select “File>Preferences>settings>Additional Boards Manager URLs” to fill the link.
Here are the steps to install the ESP32 board in Arduino IDE:
Open the Arduino IDE
Select File and then Preferences
Find the Additional Board Manager URLs field and activate the text box
Add the URL to the field
Click OK to save the changes
https://raw.githubusercontent.com/espressif/arduino-esp32/gh-pages/package_esp32_dev_index.json,
https://arduino.esp8266.com/stable/package_esp8266com_index.json,
https://espressif.github.io/arduino-esp32/package_esp32_index.json
LVGL Library: Available through the Library Manager in the Arduino IDE.
Touch Driver Library: Depending on your touchscreen (e.g., TFT_eSPI)
Configuring LVGL
LVGL Configuration: You may need to configure LVGL for your specific display and touch screen. Create a lv_conf.h file or modify the existing one in the LVGL library folder:
Set LVGL_DISPLAY_HOR_RES and LVGL_DISPLAY_VER_RES to match your display's resolution.
Configure the display and touch input settings based on your library (e.g., TFT_eSPI settings).
TFT_eSPI Configuration:
A feature rich Arduino IDE compatible graphics and fonts library for 32-bit processors. The library is targeted at 32-bit processors, it has been performance optimised for RP2040, STM32, ESP8266 and ESP32 types, other 32-bit processors may be used but will use the slower generic Arduino interface calls. The library can be loaded using the Arduino IDE's Library Manager. Direct Memory Access (DMA) can be used with the ESP32, RP2040 and STM32 processors with SPI interface displays to improve rendering performance. DMA with a parallel interface (8 and 16-bit) is only supported with the RP2040.
Go to the TFT_eSPI library folder and open the User_Setup.h file to configure the pins according to your wiring.
Wiring the TFT Display
TFT Pin ESP32 Pin
VCC 3.3V
GND GND
CS GPIO 5
RESET GPIO EN
DC/RS GPIO 17
SDI(MOSI) GPIO 23
SCK GPIO 18
LED 3.3V (or PWM pin for brightness)
Wiring the Touchscreen (e.g., TFT_espi)
Touch Pin ESP32 Pin
T_CS GPIO 16
T_IRQ GPIO 21
T_DOUT GPIO 19
T_DIN GPIO 23
T_CLK GPIO 18
4. UI Library: User Interface (e.g., UI)
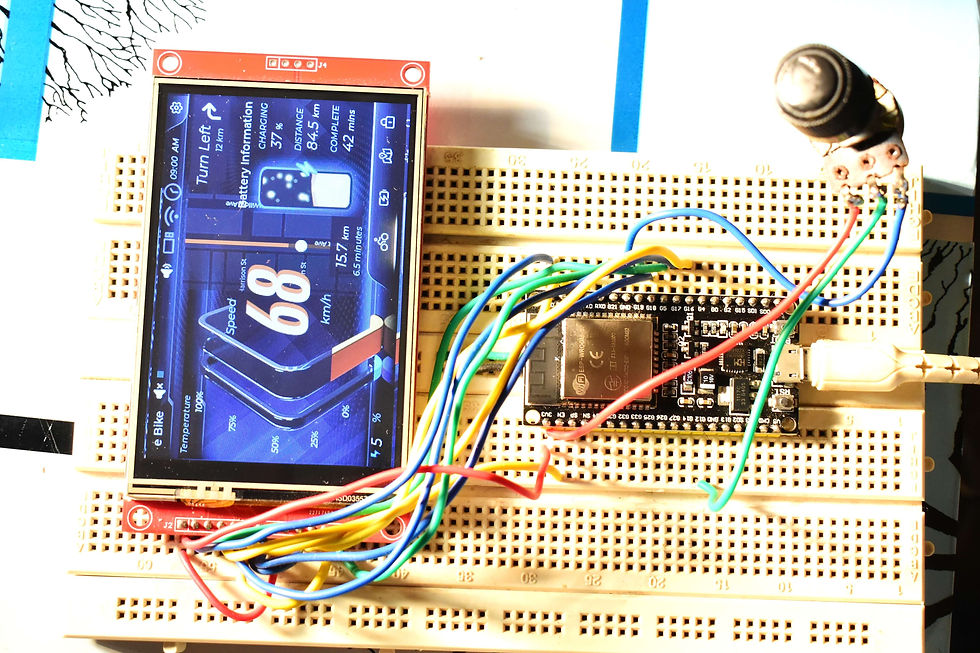
Arduino Code
Here’s a basic example to initialize the display and create a simple button using LVGL:
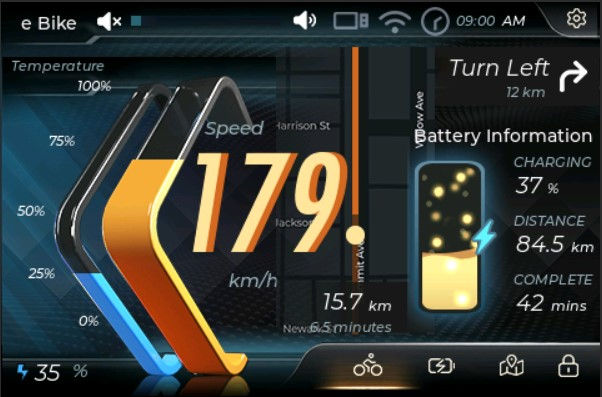
######################################################################
#include <lvgl.h>
#include <TFT_eSPI.h>
#include <ui.h>
/*Don't forget to set Sketchbook location in File/Preferences to the path of your UI project (the parent foder of this INO file)*/
/*Change to your screen resolution*/
static const uint16_t screenWidth = 480;
static const uint16_t screenHeight = 320;
static lv_disp_draw_buf_t draw_buf;
static lv_color_t buf[ screenWidth * screenHeight / 10 ];
TFT_eSPI tft = TFT_eSPI(screenWidth, screenHeight); /* TFT instance */
#if LV_USE_LOG != 0
/* Serial debugging */
void my_print(const char * buf)
{
Serial.printf(buf);
Serial.flush();
}
/* Display flushing */
void my_disp_flush( lv_disp_drv_t disp, const lv_area_t area, lv_color_t *color_p )
{
uint32_t w = ( area->x2 - area->x1 + 1 );
uint32_t h = ( area->y2 - area->y1 + 1 );
tft.startWrite();
tft.setAddrWindow( area->x1, area->y1, w, h );
tft.pushColors( ( uint16_t )&color_p->full, w h, true );
tft.endWrite();
lv_disp_flush_ready( disp );
}
/*Read the touchpad*/
void my_touchpad_read( lv_indev_drv_t indev_driver, lv_indev_data_t data )
{
uint16_t touchX = 0, touchY = 0;
bool touched = false;//tft.getTouch( &touchX, &touchY, 600 );
if( !touched )
{
data->state = LV_INDEV_STATE_REL;
}
else
{
data->state = LV_INDEV_STATE_PR;
/*Set the coordinates*/
data->point.x = touchX;
data->point.y = touchY;
Serial.print( "Data x " );
Serial.println( touchX );
Serial.print( "Data y " );
Serial.println( touchY );
}
}
void setup()
{
Serial.begin( 115200 ); /* prepare for possible serial debug */
String LVGL_Arduino = "Hello Arduino! ";
LVGL_Arduino += String('V') + lv_version_major() + "." + lv_version_minor() + "." + lv_version_patch();
Serial.println( LVGL_Arduino );
Serial.println( "I am LVGL_Arduino" );
lv_init();
#if LV_USE_LOG != 0
lv_log_register_print_cb( my_print ); /* register print function for debugging */
tft.begin(); /* TFT init */
tft.setRotation( 3 ); /* Landscape orientation, flipped */
lv_disp_draw_buf_init( &draw_buf, buf, NULL, screenWidth * screenHeight / 10 );
/*Initialize the display*/
static lv_disp_drv_t disp_drv;
lv_disp_drv_init( &disp_drv );
/*Change the following line to your display resolution*/
disp_drv.hor_res = screenWidth;
disp_drv.ver_res = screenHeight;
disp_drv.flush_cb = my_disp_flush;
disp_drv.draw_buf = &draw_buf;
lv_disp_drv_register( &disp_drv );
/*Initialize the (dummy) input device driver*/
static lv_indev_drv_t indev_drv;
lv_indev_drv_init( &indev_drv );
indev_drv.type = LV_INDEV_TYPE_POINTER;
indev_drv.read_cb = my_touchpad_read;
lv_indev_drv_register( &indev_drv );
ui_init();
Serial.println( "Setup done" );
}
void loop()
{
int rpm_value=map(analogRead(12),0,4095,0,250);
uilabel_set_property(ui_Speed_Number_1, UILABEL_PROPERTY_TEXT, String(rpm_value).c_str());
uilabel_set_property(ui_Speed_Number_2, UILABEL_PROPERTY_TEXT, String(rpm_value).c_str());
lv_slider_set_value(ui_Slider_Speed, rpm_value,LV_ANIM_ON);
int Bat_value=map(analogRead(14),0,4095,0,100);
uilabel_set_property(ui_Label_Battery_Number, UILABEL_PROPERTY_TEXT, String(Bat_value).c_str());
lv_slider_set_value(ui_Slider_Battery, Bat_value,LV_ANIM_ON);
lv_timer_handler(); /* let the GUI do its work */
delay(5);
}
######################################################################
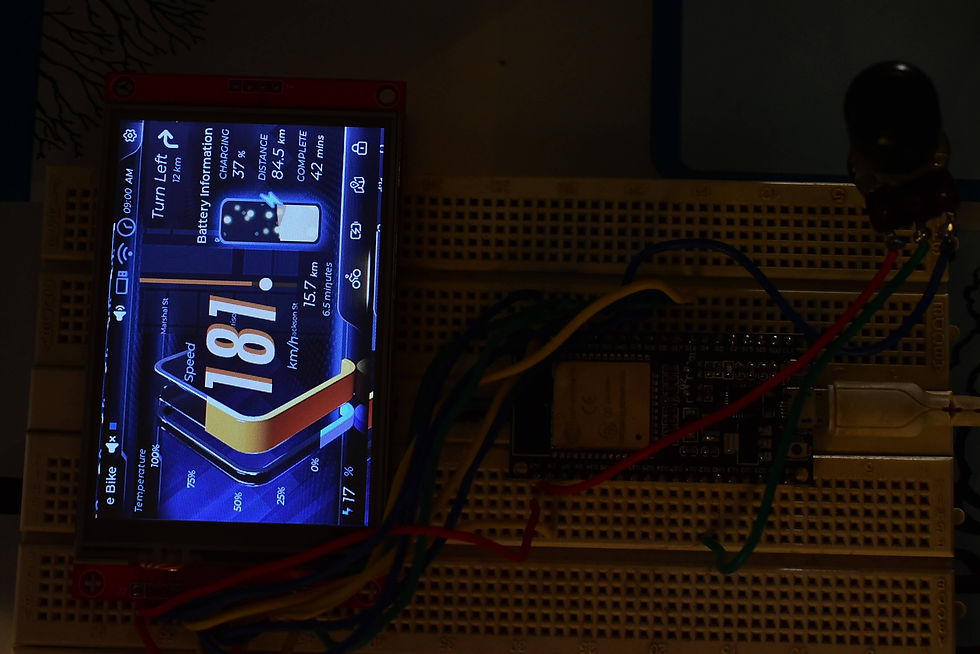
Final Steps
Upload the code to your ESP32 using the Arduino IDE.
Open the Serial Monitor to check for any debug messages.
Interact with the touchscreen to see your UI in action!
Tips
Adjust the pin assignments based on your wiring.
Explore LVGL's extensive documentation for more UI elements and functionalities.
Use LVGL's built-in tools to design and customize your interfaces.
This should give you a solid start on using LVGL with an ESP32 and a TFT LCD touchscreen. Happy coding!
Commentaires