In this project, I will show you how to build a simple Light Activated Switch Circuit using LDR. Using this circuit, an electrical device or an appliance like a street light or a fan for example, can be controlled based on the intensity of the light near the circuit. In this to build an IoT system using an ESP8266, myDevices Cayenne, and MQTT. In more detail, this IoT tutorial discovers how to use an ESP8266 to send data to Cayenne using the MQTT protocol. Moreover, this ESP8266 MQTT project investigates how to use MQTT to control remote peripheral devices using a web interface. This is a complete step-by-step tutorial on building an IoT system using Cayenne and ESP.

On the other hand, Cayenne is an IoT cloud platform that provides several cloud services, such as:
Data visualization
IoT cloud
Alerts
Scheduling Events
We will focus our attention on data visualization and on the IoT cloud services.
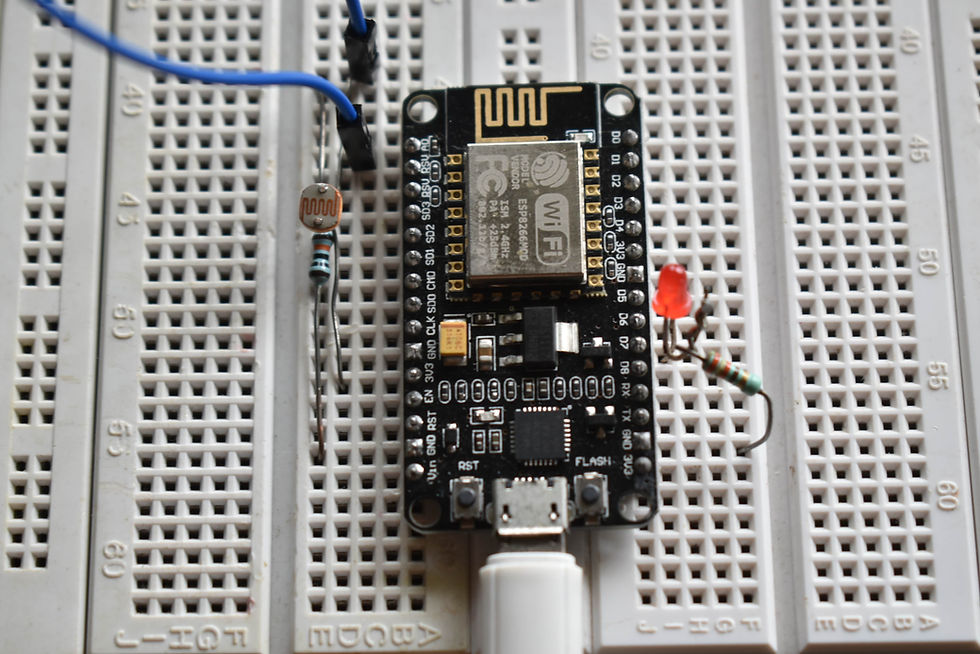
Cayenne
Cayenne IoT Platform accelerates the development of IoT-based solutions, including quick design, prototyping and other commercialized projects. It is a drag-and-drop IoT project builder that can help developers build complete, ready-to-use IoT solutions with little to no coding.
Cayenne IoT Platform contains a vast catalog of certified IoT-ready devices and connectivity options. This allows users to easily add any device to the library utilizing MQTT API. All devices in Cayenne are interoperable and benefit from features such as rules engine, asset tracking, remote monitoring and control, and tools to visualize real-time and historical data.
MQTT is a lightweight messaging protocol for sensors and mobile devices.
NodeMCU
NodeMCU ESP8266-12E MCU is a development board with one analogue and many general-purpose input output (GPIO) pins. It has 4MB flash memory, and can operate at a default clock frequency of 80MHz. In this project, Analog pin A0 of NodeMCU is used to read Voltage in Between LDR and Series Resister.
LDR
Light dependent resistors, LDRs, or a photoresistor is a passive component that decreases resistance with respect to receiving luminosity on the component's sensitive surface. The resistance of a photoresistor decreases with increase in incident light intensity; in other words, it exhibits photoconductivity.
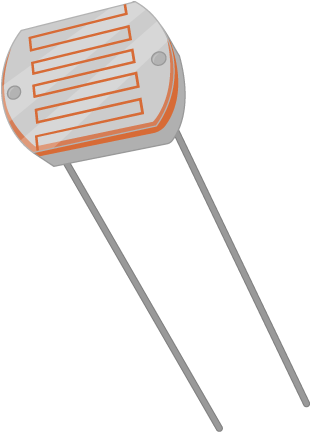
Principle behind the Circuit
The main principle of this circuit is based on the working of the LDR Sensor i.e. the Light Dependent Resistor and to switch ON or OFF the light based on the intensity of illumination.
Theory of the light dependent resistor, it will have a high resistance in darkness and low resistance in the presence of light.
A typical light dependent resistor, LDR / photoresistor specification may be:
Example Photo Resistors Spec parameters Example below.
Max power dissipation - 200mWMax
voltage @ 0 lux - 200V
Peak wavelength - 600nmMin.
resistance @ 10lux - 1.8kΩMax.
resistance @ 10lux - 4.5kΩTyp.
resistance @ 100lux - 0.7kΩ
Dark resistance after 1 sec - 0.03MΩ
Dark resistance after 5 sec - 0.25MΩ
Installing the ESP8266_Arduino_Library
Download the Cayenne-MQTT-ESP-master library from this link.
Click on Add ZIP Library and add Cayenne-MQTT-ESP-master zip file, or directly copy the folder (Cayenne-MQTT-ESP-master) and paste it in Libraries folder of Arduino IDE.
After installing the library, go to your Arduino IDE. Make sure you have the Nodemcu 1.0 ESP-12E board selected, and then, Copy and Paste code in Arduino IDE.
Circuit Diagram
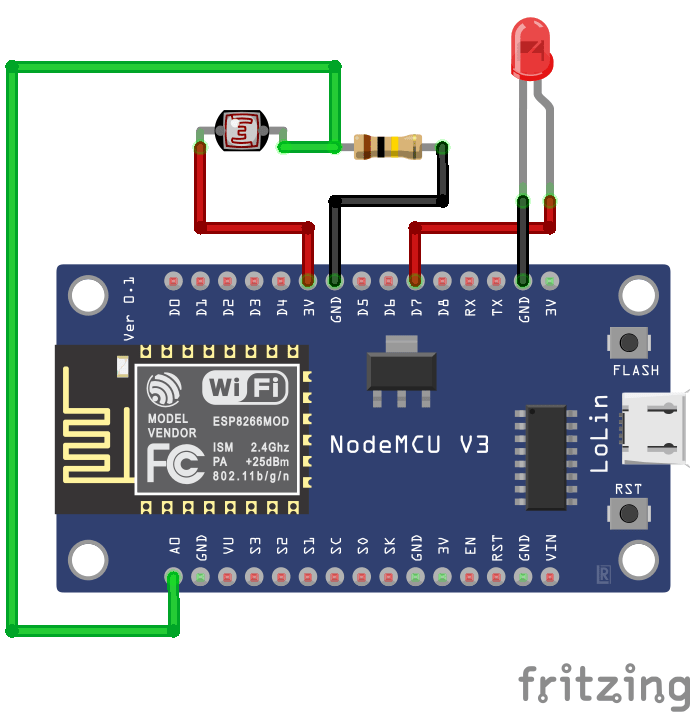
Subscribe and Download code.
Arduino code
#include <CayenneMQTTESP8266.h>
#include <ESP8266WiFi.h>
char ssid[] = "SSID"; //YOUR SSID
char password[]="PASSWORD"; // YOUR PASSWORD
char username[] = "2031dd30-5414-11eb-b767-3f1a8f1211ba"; ////YOURs
char mqtt_password[] = "f2ce829d98df3a768328ac6936eae9fd47d28289"; ////YOURs
char client_id[] = "3d65e860-5414-11eb-a2e4-b32ea624e442"; // //YOURs
int ldr = A0;
int ldr_data;
void setup()
{
Serial.begin(9600);
Cayenne.begin(username,mqtt_password,client_id,ssid,password);
pinMode(A0,INPUT);
}
void loop()
{
Cayenne.loop();
ldr_data = analogRead(ldr);
Serial.println(ldr_data);
Cayenne.virtualWrite(2, ldr_data);
}
Hardware interfacing with Cayenne IoT platform
Click on Add new and then Device/Widget in Settings, Add New Device here and select Generic ESP8266 for in this project.
Configure device Generic ESP8266, MQTT username, password and client ID from Create App
Paste these respective details under username, password and client ID in Arduino source code , along with your Wi-Fi name and password.
After successfully compiling and uploading the code to NodeMCU, You will see ESP8266 connected to Wi-Fi. After the connection is established, the previous page is automatically updated on Cayenne. A new dashboard opens in the browser. Cayenne generates an ID and a device icon for your device.
Click on Custom Widgets and then value, and populate all fields . The channel number should be 1. (Make sure the channel number is same as in code.) Now, click on Add Widget.
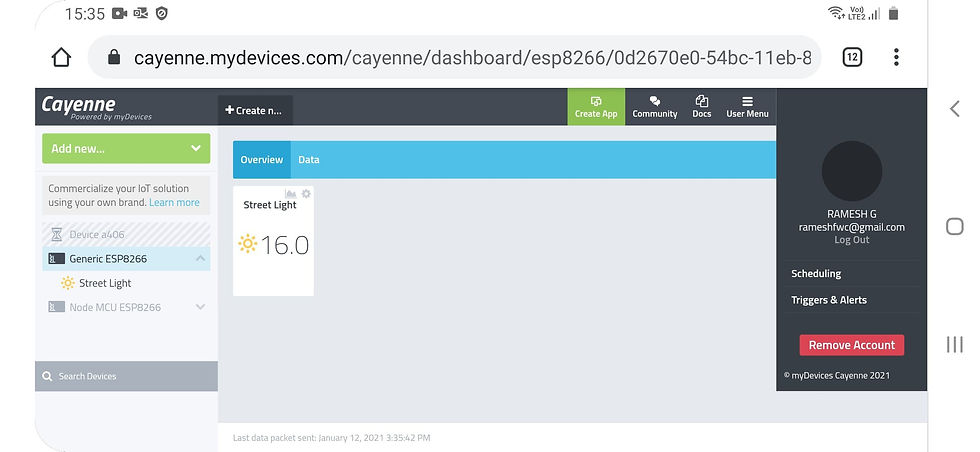
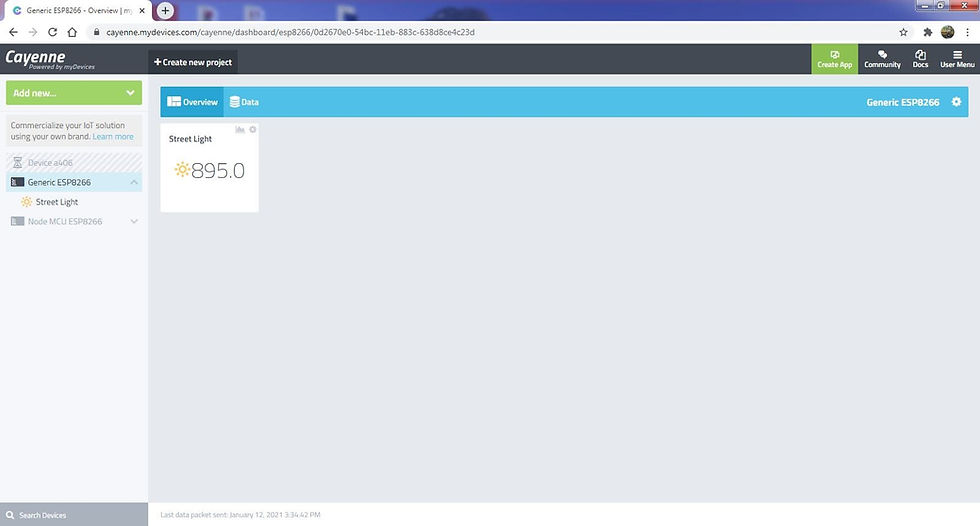
Graphical representation by click Graph Icon.
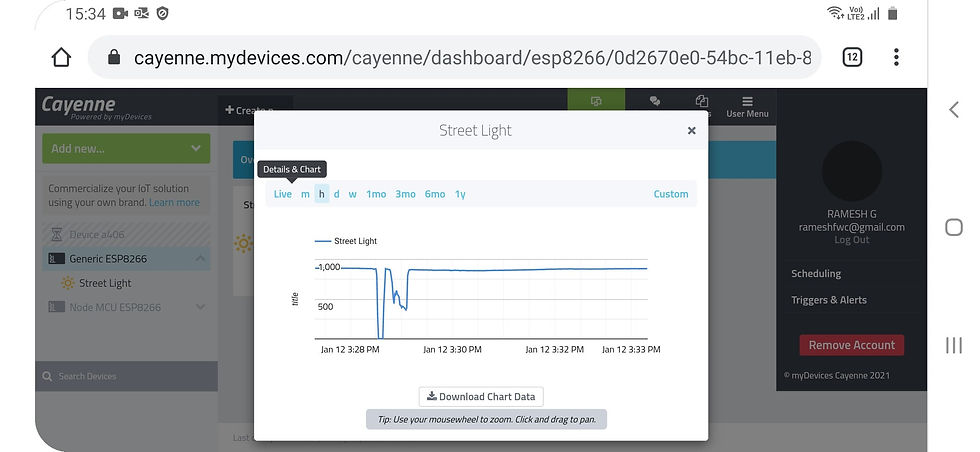
Comentários